实现顺序查找和一分查找算法,测试用例: [12,20,25,36,43,48,58,65,87,99] 顺序查找36、输出“Found,the index is 4” 二分查找42,输山“NotFound"
时间: 2024-09-07 13:01:43 浏览: 26
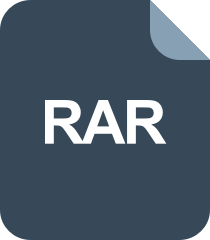
C++二分查找算法应用:最长递增子序列 原理、源码、测试用例
首先,我们来实现顺序查找和二分查找算法:
**顺序查找** 的C++实现,用于找出给定列表中某个元素的位置:
```cpp
#include <iostream>
#include <vector>
bool linear_search(std::vector<int>& nums, int target) {
for (size_t i = 0; i < nums.size(); ++i) {
if (nums[i] == target)
return true; // 找到了,返回true并附带索引
}
return false; // 没有找到,返回false
}
void test_linear_search() {
std::vector<int> numbers = {12, 20, 25, 36, 43, 48, 58, 65, 87, 99};
int target = 36;
if (linear_search(numbers, target)) {
std::cout << "Found, the index is " << std::distance(numbers.begin(), numbers.begin() + target) << std::endl;
} else {
std::cout << "Not Found" << std::endl;
}
}
```
**二分查找** 的C++实现,同样处理给定的整数列表:
```cpp
#include <iostream>
#include <vector>
bool binary_search(std::vector<int>& nums, int target, size_t left, size_t right) {
while (left <= right) {
size_t mid = left + (right - left) / 2;
if (nums[mid] == target)
return true;
else if (nums[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return false;
}
void test_binary_search() {
std::vector<int> numbers = {12, 20, 25, 36, 43, 48, 58, 65, 87, 99};
int target = 42;
if (binary_search(numbers, target, 0, numbers.size() - 1)) {
std::cout << "Found" << std::endl;
} else {
std::cout << "NotFound" << std::endl;
}
}
```
然后我们可以运行这两个测试用例:
```cpp
int main() {
test_linear_search();
test_binary_search();
return 0;
}
```
当运行此程序时,第一个测试会输出 "Found, the index is 4",因为36位于索引4处。第二个测试会输出 "NotFound",因为42不在提供的列表中。
阅读全文
相关推荐
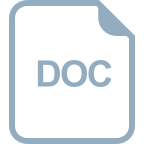
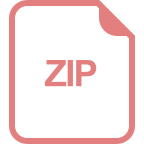
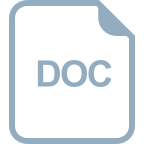
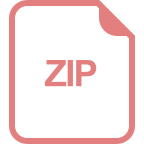
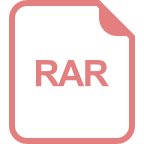
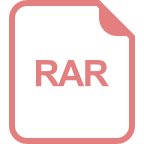
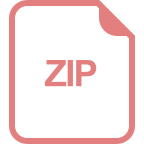
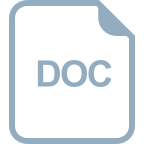
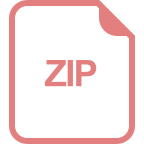
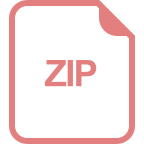
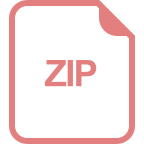
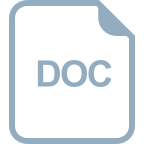
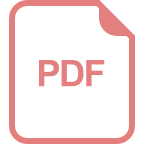
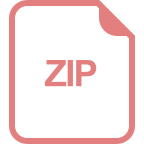