利用Python画一朵花
时间: 2023-04-09 22:02:53 浏览: 113
我可以回答这个问题。要利用Python画一朵花,可以使用Python的绘图库matplotlib。以下是一个简单的示例代码:
```
import matplotlib.pyplot as plt
import numpy as np
# 生成花瓣的坐标
t = np.linspace(0, 2*np.pi, 100)
x = np.sin(t) * (np.exp(np.cos(t)) - 2*np.cos(4*t) - np.sin(t/12)**5)
y = np.cos(t) * (np.exp(np.cos(t)) - 2*np.cos(4*t) - np.sin(t/12)**5)
# 绘制花瓣
plt.plot(x, y)
# 显示图像
plt.show()
```
这段代码会生成一朵花的图像。你可以根据需要调整代码中的参数,来绘制不同形状的花朵。
相关问题
用python画出一朵玫瑰花
### 回答1:
你可以使用Python的Matplotlib库来实现,具体步骤如下:
1. 导入Matplotlib库:import matplotlib.pyplot as plt
2. 定义绘图参数:x = np.arange(0, 2*np.pi, 0.01)
3. 绘制玫瑰花:y = np.sin(x)
4. 显示绘图:plt.plot(x, y)
5. 保存绘图:plt.savefig('rose.png')
### 回答2:
要用Python画出一朵玫瑰花,可以使用turtle模块来实现。首先,我们需要导入相应的库:
```python
import turtle
```
然后,创建一个绘图窗口,并设置窗口的大小和背景颜色:
```python
window = turtle.Screen()
window.bgcolor("white")
```
接下来,我们可以定义一个函数来绘制玫瑰花的一个花瓣,函数的形式如下:
```python
def draw_petal():
for i in range(18):
turtle.forward(50)
turtle.left(45)
turtle.forward(50)
turtle.left(135)
```
在函数内部,使用for循环语句来控制海龟绘制出玫瑰花的一个花瓣。其中,turtle.forward()函数用来向前移动一定的距离,turtle.left()函数用来左转一定的角度。
接下来,我们可以调用这个函数绘制出玫瑰花的所有花瓣。可以使用for循环语句来控制绘制的次数,从而画出完整的玫瑰花:
```python
for i in range(12):
draw_petal()
turtle.left(30)
```
最后,记得在结束前调用turtle.done()函数来显示绘图结果:
```python
turtle.done()
```
以上是用Python绘制玫瑰花的一个简单实现。根据需要,还可以设置海龟的颜色、线宽等属性来美化玫瑰花的效果。
### 回答3:
要用Python画出一朵玫瑰花,可以利用turtle库来实现。下面是实现该功能的代码:
```python
import turtle
# 设置画布和画笔的颜色
canvas = turtle.Screen()
canvas.bgcolor("white")
brush = turtle.Turtle()
brush.color("red")
# 控制画笔的形状
brush.shape("circle")
# 画出花朵的轮廓
for _ in range(6):
brush.circle(100, 60)
brush.penup()
brush.right(120)
brush.pendown()
# 绘制花瓣
for _ in range(6):
brush.penup()
brush.goto(0, 0)
brush.pendown()
brush.right(60)
brush.circle(100, 120)
# 绘制花蕊
brush.color("yellow")
brush.penup()
brush.goto(0, -40)
brush.pendown()
brush.circle(20)
# 完成绘制
turtle.done()
```
以上代码使用turtle库来实现花的绘制,首先设置了画布和画笔的颜色。然后通过循环控制画笔画出玫瑰花的轮廓,每次画60度的圆弧,当画完一次后,将画笔向右旋转120度,并将画笔移起来再放下。接着用循环绘制6个花瓣,每次旋转60度,并画出120度的圆弧,再次画完后将画笔移起来,重置位置。最后,用黄色画笔绘制花蕊,并完成绘制。
利用python代码画出一幅特别好看的玫瑰花束
好的,以下是可以绘制出一幅漂亮的玫瑰花束的 Python 代码:
```
import turtle
def draw_flower(t, petal_count, petal_size, petal_angle):
for i in range(petal_count):
# Draw the petal
t.circle(petal_size, petal_angle)
# Turn to draw the next petal
t.left(180 - petal_angle)
t.circle(petal_size, petal_angle)
# Turn to draw the next petal
t.left(180 - petal_angle + 360 / petal_count)
# Move to center to draw the stigma
t.penup()
t.goto(0, 0)
t.pendown()
# Draw the stigma
t.dot(petal_size // 8)
# Setup the canvas
t = turtle.Turtle()
t.speed(0)
t.width(3)
t.getscreen().bgcolor('#F1948A')
# Draw the flower
draw_flower(t, 10, 100, 20)
# Hide the turtle and display the canvas
t.hideturtle()
turtle.done()
```
这段代码使用 Python 的 `turtle` 模块来绘制一朵玫瑰花。具体来说,它定义了一个函数 `draw_flower`,该函数接受四个参数:`petal_count` 表示花瓣数量,`petal_size` 表示花瓣大小,`petal_angle` 表示相邻花瓣之间的角度。然后,它使用 `turtle` 对象 `t` 来绘制花朵,具体的绘制方法是循环往复绘制花瓣,直到所有花瓣都绘制完成。最后,在花芯处添加了一个小圆点作为花蕊。
相关推荐
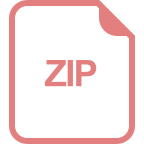
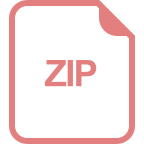
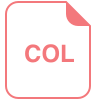
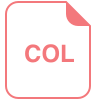
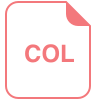
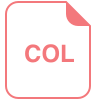
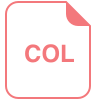






