如何在编程中正确地提取字符串的一部分
时间: 2024-11-20 08:41:33 浏览: 5
在编程中,正确地提取字符串的一部分通常涉及到字符串操作或索引技巧。这里有几个常见的方法:
1. **基于索引**:你可以使用字符串的`[]`索引来获取子串。例如,在Python中,`my_string[start:end]`会返回从第`start`位到第`end - 1`位的所有字符(非包含结束位置)。如果省略结束位置,则默认提取到字符串末尾。
```python
s = "Hello, World!"
substring = s[7:12] # 输出 "World"
```
2. **切片**:类似上面的索引,许多语言支持切片语法,如Java、JavaScript等。
```java
String str = "Hello, Java!";
String subStr = str.substring(7, 12); // 输出 "Java"
```
3. **正则表达式**:如果你需要按照模式匹配提取部分,可以使用正则表达式库。比如在Python中,`re`模块的`search()`或`findall()`函数可以找到匹配的子串。
```python
import re
text = "My email is example@example.com"
match = re.search(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b', text)
email = match.group() if match else None; # 提取电子邮件地址
```
4. **字符串处理函数**:有些语言有内置的函数或方法用于分割字符串,如Python的`split()`或JavaScript的`slice()`,它们可以根据特定分隔符或长度切割字符串。
```python
words = "this.is.a.sample".split('.') # 输出 ["this", "is", "a", "sample"]
```
阅读全文
相关推荐
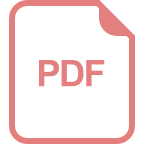
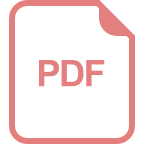
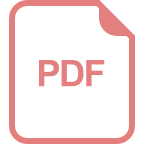
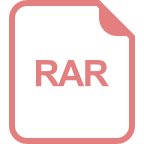
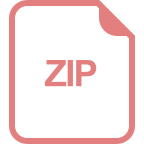
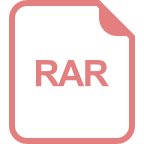
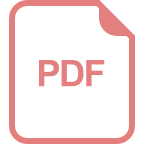
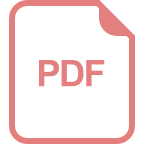
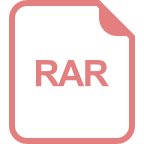
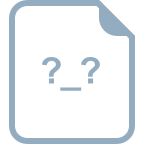
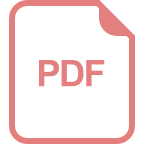
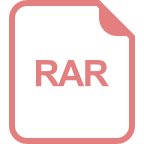
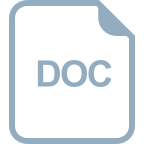
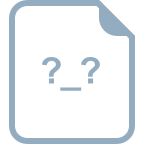
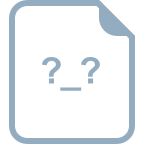
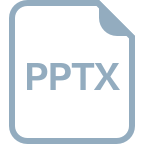
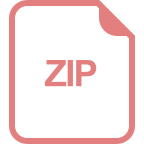
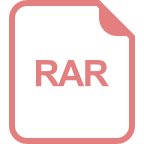