@GetMapping("/check") public Result check(){ long nowtime = new Date().getTime(); if ((nowtime - staretime)/1000 > 1800) userid = -1; if (userid == -1){ return Result.error(); } return Result.success(); }
时间: 2024-02-14 11:22:43 浏览: 22
这是一个 Java Spring Boot 框架中的一个控制器(Controller)方法,使用了 GetMapping 注解,映射了 "/check" 路径。该方法的作用是检查用户是否登录过期。
方法中定义了一个当前时间的变量 nowtime,使用了 new Date().getTime() 方法获取当前时间的毫秒数。然后通过计算当前时间和用户登录时间的差值,判断用户是否登录超时(1800秒即30分钟)。若超时,将用户id设置为-1,表示未登录,返回 Result.error();若未超时,返回 Result.success()。
需要注意的是,代码中的 staretime 变量未定义,可能是在其他地方定义的全局变量。此外,代码中的 userid 变量也未定义,需要在其他地方定义或传入该方法中。
相关问题
public class UserController { private static Integer userid = -1; long staretime = 0; @Autowired IUserService iUserService; @GetMapping("/selectone") public Result selectone(){ User user = iUserService.selectuser(userid); if (user == null) return Result.error(); return Result.success(user); } @GetMapping("/check") public Result check(){ long nowtime = new Date().getTime(); if ((nowtime - staretime)/1000 > 1800) userid = -1; if (userid == -1){ return Result.error(); } return Result.success(); } @PostMapping("/login") public Result login(@RequestBody User user){ Integer login = iUserService.login(user); userid = login; staretime = new Date().getTime(); if (userid == -1){ return Result.error(); }else { return Result.success(userid); } } @GetMapping("/serach") public Result serach(@RequestParam String serachinput){ List<User> serachbyusername = iUserService.serachbyusername(serachinput); if (!serachbyusername.isEmpty()){ return Result.success(serachbyusername); } return Result.error(); } @PostMapping("/register") public Result register(@RequestBody User user){ boolean register = iUserService.register(user); if (register){ return Result.success(); }else{ return Result.error(); } } @GetMapping("/display") public Result display(){ List<User> display = iUserService.display(); if (display.isEmpty()){ return Result.error(); }else { return Result.success(display); } } @PostMapping("/edit") public Result edit(@RequestBody User user){ boolean res = iUserService.updata(user); if (res) { return Result.success(); } return Result.error(); } @GetMapping("/delete") public Result delete(@RequestParam String userid){ int integer = Integer.parseInt(userid); boolean res = iUserService.delete(integer); if (res) { return Result.success(); } return Result.error(); } }
这是一个 Java Spring Boot 框架中的用户控制器(UserController)类,包含了多个请求处理方法,对应了不同的用户操作。
在该类中,定义了一个静态的 userid 变量,用于保存当前登录用户的id。还有一个 staretime 变量,用于保存用户登录的时间。
该类使用了 @Autowired 注解,注入了 IUserService 接口的实现类,以便调用该接口提供的服务。
在该类中,定义了多个请求处理方法,包括:
- selectone():根据当前登录用户的id,查询用户信息并返回。
- check():检查用户是否登录过期。
- login():用户登录,验证用户信息,并将登录用户的id保存到 userid 变量中。
- serach():根据输入的关键字,搜索匹配的用户信息并返回。
- register():用户注册,将新用户信息保存到数据库中。
- display():显示所有用户信息。
- edit():编辑用户信息,更新用户信息到数据库中。
- delete():删除指定用户信息。
这些方法都返回 Result 对象,该对象包含一个状态码和一个数据对象,用于表示请求处理的结果。其中,成功的状态码为0,失败的状态码为1。
public class UserController { private static Integer userid = -1; long staretime = 0; @Autowired IUserService iUserService; @GetMapping("/selectone") public Result selectone(){ User user = iUserService.selectuser(userid); if (user == null) return Result.error(); return Result.success(user); }
这是一个Spring Boot的UserController类,其中包含一个selectone方法。该方法使用@GetMapping注释标记,表示它将处理HTTP GET请求。当该方法被调用时,它将从数据库中获取一个用户对象,并将其作为JSON响应返回。该方法还使用@Autowired注释将IUserService接口的实现注入到UserController中。另外,该类还包含了一个静态的userid变量和一个staretime变量,但是这两个变量没有在selectone方法中使用。
相关推荐
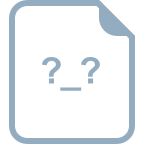
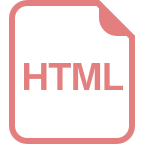












