选择一幅彩色图像(如使用经典的Lena图像),Python编程实现基于全局阈值和自适应阈值的图像分割算法
时间: 2024-01-21 15:16:06 浏览: 26
好的,我可以为你提供代码实现。
首先,我们需要导入必要的库和图像:
```python
import cv2
import numpy as np
# 读取彩色图像
img = cv2.imread('lena.png')
```
接着,我们可以使用全局阈值方法进行图像分割。这里我们选择使用Otsu算法来确定阈值:
```python
# 全局阈值分割
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh1 = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
```
然后,我们可以使用自适应阈值方法进行图像分割。这里我们选择使用自适应高斯阈值方法:
```python
# 自适应阈值分割
thresh2 = cv2.adaptiveThreshold(gray, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, 11, 2)
```
最后,我们将分割结果保存为图像:
```python
# 保存分割结果
cv2.imwrite('global_threshold.png', thresh1)
cv2.imwrite('adaptive_threshold.png', thresh2)
```
完整代码如下:
```python
import cv2
import numpy as np
# 读取彩色图像
img = cv2.imread('lena.png')
# 全局阈值分割
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh1 = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
# 自适应阈值分割
thresh2 = cv2.adaptiveThreshold(gray, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, 11, 2)
# 保存分割结果
cv2.imwrite('global_threshold.png', thresh1)
cv2.imwrite('adaptive_threshold.png', thresh2)
```
运行后,会生成两张分割后的图像:`global_threshold.png`和`adaptive_threshold.png`。
相关推荐
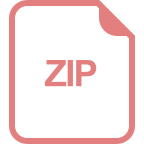
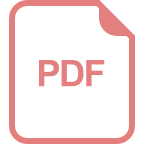














