vue3根据父元素的ref获取子元素
时间: 2024-12-24 17:41:01 浏览: 4
在Vue3中,如果你想要根据父元素的`ref`来获取其子元素,你可以这样做:
1. 首先,在你的组件模板或某个计算属性中,定义一个父元素的`ref`,例如:
```html
<template>
<div ref="parentRef">
<!-- 子元素 -->
<child-component :key="index"></child-component>
</div>
</template>
```
这里假设`parentRef`是一个`ref`,并绑定了到一个`div`元素。
2. 然后,在你的组件的script部分,可以使用`.el`属性访问这个`ref`的元素实例:
```javascript
<script setup>
import ChildComponent from './ChildComponent.vue';
const parentRef = ref(null);
let childElements;
onMounted(() => {
childElements = parentRef.value.querySelectorAll('child-component'); // 获取所有的子元素
});
</script>
```
这样,`childElements`变量就会包含所有`child-component`的Vue实例数组,你可以通过索引来操作它们。
相关问题
vue3根据父元素的ref获取第3个子元素
在Vue3中,你可以通过`ref`属性创建一个引用到DOM元素,并结合数组索引来访问其子元素。假设你有一个父组件,它有三个子元素,你可以这样做:
```javascript
<template>
<div ref="parentRef">
<child-component v-for="(item, index) in items" :key="index" :childProp="item"></child-component>
</div>
</template>
<script>
export default {
data() {
return {
items: [
// 这里是你需要的数据
{ childProp: '子元素1' },
{ childProp: '子元素2' },
{ childProp: '子元素3' } // 需要获取的就是这个
],
parentRef: null,
};
},
mounted() {
this.parentRef = this.$refs.parentRef; // 获取父元素ref
const thirdChild = this.parentRef.children[2]; // 访问第3个子元素
console.log(thirdChild); // 打印第三个子元素
}
};
</script>
```
这里,我们首先在模板中设置了`ref="parentRef"`,然后在`mounted()`钩子函数中,我们将父元素的引用赋值给`parentRef`变量。最后,通过`children`数组的索引`2`获取到了第3个子元素。
vue3,ref,子模板重新设置父元素
Vue3中的`ref`是响应式数据容器,它提供了一个类似React中的状态管理功能。当你创建一个`ref`实例并给它赋值时,它的值会自动绑定到视图上,并且当值改变时,视图会自动更新。
关于你在子组件模板中更新父组件的`ref`,通常是在子组件需要修改其从父组件传递过来的状态时。例如:
```html
<template>
<div>
<!-- 子组件内部操作 -->
<button @click="updateParentValue">更新父值</button>
<p v-text="parentValue"></p>
</div>
</template>
<script>
export default {
methods: {
updateParentValue() {
this.$emit('update:parentValue', newValue); // 使用$emit触发自定义事件
// 或者直接修改父ref的值,如果父组件已经使用setup生命周期钩子
// this.$parent.myRef.value = newValue;
}
}
}
</script>
```
在这个例子中,`updateParentValue`方法通过`$emit`将新的值发送回父组件,父组件监听这个事件并在接收到新值后更新对应的`ref`。注意,这里假设父组件通过`v-model`或者`prop`接收`ref`的值,并且设置了`sync`修饰符以实现在子组件更改时同步更新。
阅读全文
相关推荐
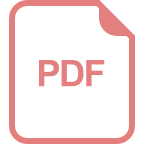
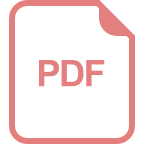
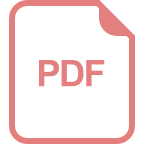













