高精度string加法模板 考虑负数 c++
时间: 2024-10-21 12:11:44 浏览: 32
高精度字符串加法在C++中通常通过动态数组或者`std::vector`存储大整数,并实现自定义的加法操作。对于考虑负数的情况,我们需要处理正数加负数、两个负数以及不同位数的相加。以下是一个简单的模板,使用了`std::vector`来存储字符数组代表的大数:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
// 定义大数结构体
struct BigInt {
std::vector<char> digits;
bool isNegative;
// 构造函数、获取长度等基础操作...
};
// 自定义加法函数
BigInt add(BigInt a, BigInt b) {
if (a.isNegative != b.isNegative) { // 判断是否一正一负
BigInt result = a.isNegative ? -b : a; // 取绝对值
return add(result, b);
}
BigInt result;
result.isNegative = a.isNegative; // 结果的负号取决于原始数值
size_t maxLen = std::max(a.digits.size(), b.digits.size());
result.digits.resize(maxLen + 1); // 预留足够的空间
// 数字从右向左逐位相加
for (size_t i = maxLen; i > 0; --i) {
char sum = '0' + (a.digits[i - 1] - '0' + b.digits[i - 1] - '0') % 10; // 相加并考虑进位
result.digits[i] = static_cast<char>(sum);
if (result.digits[i - 1] >= '9' && sum != '0') { // 检查是否需要进位
result.digits[i - 1]++;
if (i == maxLen) { // 如果是最高位还需要进位,则处理最高位
if (result.digits[0] != '0' || a.isNegative) {
result.digits[0] = '1';
result.isNegative = true; // 进位导致最高位变正
} else {
result.isNegative = false;
}
}
}
}
return result;
}
// 示例
int main() {
BigInt num1 = {"12345", true}; // 负数12345
BigInt num2 = {"67890", false}; // 正数67890
BigInt sum = add(num1, num2);
// 输出结果
// ...
return 0;
}
```
阅读全文
相关推荐
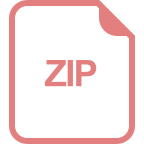
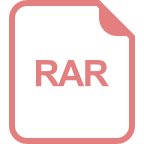
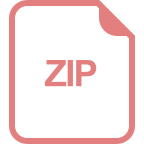

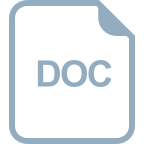
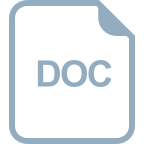
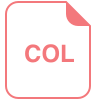











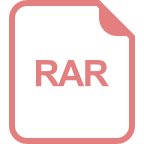
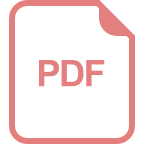