c语言字符串的排列 描述:给定一个字符串,返回该字符串按字典序排列的所有可能组合。 示例:输入:"abc",输出:["abc", "acb", "bac", "bca", "cab", "cba"
时间: 2024-06-23 09:02:18 浏览: 7
在C语言中,处理字符串的所有可能排列通常涉及到回溯算法或递归,因为字符串的排列是一个典型的全排列问题。下面是一个简单的递归方法来生成字符串的所有可能排列:
```c
#include <stdio.h>
#include <string.h>
void permute(char *str, int l, int r) {
if (l == r)
printf("%s\n", str);
else {
for (int i = l; i <= r; i++) {
char temp = str[l];
str[l] = str[i];
str[i] = temp;
permute(str, l + 1, r);
// 回溯:恢复当前状态
temp = str[l];
str[l] = str[i];
str[i] = temp;
}
}
}
int main() {
char str[] = "abc";
int n = strlen(str);
permute(str, 0, n - 1);
return 0;
}
```
在这个代码中,`permute` 函数是一个递归函数,它会将第一个字符与剩余字符的每个位置进行交换,然后对剩余部分(`l + 1` 到 `r`)进行递归调用。当字符串只剩下1个字符时,就直接打印出来,这就是一个排列。递归过程中通过临时变量在每次循环后恢复原字符串状态,实现了回溯。
相关推荐
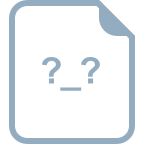














