对不规则边界最小二乘法拟合圆python
时间: 2024-03-21 16:36:34 浏览: 11
对不规则边界最小二乘法拟合圆是一种在给定一组不规则边界点的情况下,通过最小化拟合误差来找到最佳拟合圆的方法。在Python中,可以使用scipy库中的optimize模块来实现该方法。
以下是对不规则边界最小二乘法拟合圆的步骤:
1. 收集不规则边界点的坐标数据。
2. 导入必要的库:`import numpy as np`和`from scipy import optimize`
3. 定义一个函数来计算拟合误差,例如使用点到圆心的距离之和作为误差度量。
4. 定义一个函数来拟合圆,该函数将圆心坐标和半径作为参数,并返回拟合误差。
5. 使用optimize模块中的`minimize`函数来最小化拟合误差,得到最佳拟合圆的参数。
6. 根据最佳拟合圆的参数绘制拟合结果。
下面是一个示例代码:
```python
import numpy as np
from scipy import optimize
# 收集不规则边界点的坐标数据
points = np.array([[x1, y1], [x2, y2], ...])
# 定义计算拟合误差的函数
def error_function(params):
cx, cy, r = params
distances = np.sqrt((points[:, 0] - cx)**2 + (points[:, 1] - cy)**2)
return np.sum((distances - r)**2)
# 定义拟合圆的函数
def fit_circle(params):
cx, cy, r = params
return error_function(params)
# 使用最小二乘法拟合圆
initial_guess = [initial_cx, initial_cy, initial_r] # 初始猜测值
result = optimize.minimize(fit_circle, initial_guess)
# 获取最佳拟合圆的参数
best_params = result.x
best_cx, best_cy, best_r = best_params
# 绘制拟合结果
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.scatter(points[:, 0], points[:, 1], color='blue', label='Points')
circle = plt.Circle((best_cx, best_cy), best_r, color='red', fill=False, label='Best Fit Circle')
ax.add_artist(circle)
ax.set_aspect('equal')
plt.legend()
plt.show()
```
相关推荐
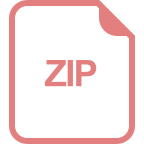
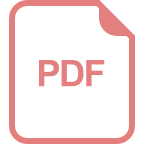














