~MyClass() { // ERROR **********found********** delete array[i]; }
时间: 2023-09-09 20:14:22 浏览: 58
在这段代码中,错误是`delete array[i]`。应该使用`delete[] array[i]`来释放数组元素的内存,因为`array`是一个指向数组的指针。正确的写法应该是:
```cpp
~MyClass() {
for (int i = 0; i < size; i++) {
delete array[i];
}
}
```
这样才能正确释放每个数组元素的内存。
相关问题
**kwargs在类里面
`**kwargs` 是一种特殊的参数语法,它在 Python 类的方法定义中用于接收不定数量的关键字参数。当你不确定或不关心方法将接收到哪些关键字参数时,可以使用这种方式。这些关键字参数会被收集到一个字典中,允许你在方法内部根据实际传入的参数动态处理。
在类的方法中,`**kwargs` 的样子通常像这样:
```python
class MyClass:
def my_method(self, param1, param2, **kwargs):
# 这里你可以访问和使用 kwargs 中的所有键值对
for key, value in kwargs.items():
print(f"Received keyword argument: {key} = {value}")
```
当你调用这个方法时,可以传递任意数量的关键字参数,例如:
```python
instance = MyClass()
instance.my_method(param1="value1", param2="value2", extra_param="additional_value")
```
全局函数运算符重载*C++
全局函数运算符重载是指在C++中通过定义全局函数来重载运算符。其中,运算符重载是指为已有的运算符重新定义一种新的使用方式或含义。在这种方式下,我们可以对自定义的数据类型使用内置运算符。
以重载乘法运算符为例,假设我们要对自定义的类对象进行乘法运算,可以通过定义一个名为operator*的全局函数来实现运算符重载。该函数的参数为两个类对象,返回值为相应的结果。
例如:
```
class MyClass{
private:
int num;
public:
MyClass(int n):num(n){}
MyClass operator*(const MyClass& obj){
return MyClass(num*obj.num);
}
};
MyClass a(10),b(20),c;
c = a*b;
```
在上述代码中,我们定义了一个MyClass类,重载了乘法运算符*。在使用时,我们可以通过a*b的形式来实现两个类对象的相乘操作。
相关推荐
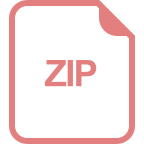
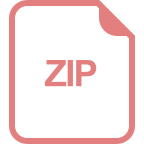
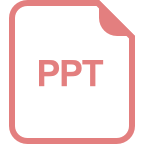













