opencv 彩色图像赋值
时间: 2024-10-04 09:03:13 浏览: 25
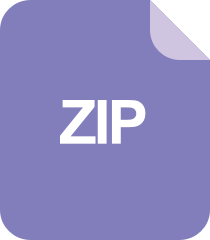
xiangsu.zip_c++像素_图像赋值
在OpenCV中,彩色图像通常是以BGR(Blue、Green、Red)颜色空间存储的,如果你想对彩色图像的某个部分赋予新的颜色,你可以使用`cv::putpixel()`函数或者直接进行像素级别的操作。下面是一个基本的例子:
```cpp
#include <opencv2/opencv.hpp>
cv::Mat original_image; // 假设已经读取了一个BGR格式的彩色图像
// 选择一个像素点 (x, y) 并赋值
cv::Vec3b new_color(255, 0, 0); // 新的颜色,例如红色
int x = 100;
int y = 100;
original_image.at<cv::Vec3b>(y, x) = new_color;
// 或者使用 putpixel 函数
cv::putpixel(original_image, x, y, new_color);
imshow("Colored Image", original_image);
waitKey();
```
在这个例子中,我们选择了原图中的坐标 (x, y),然后将该位置的像素颜色设置为红色(B=255, G=0, R=0)。注意,OpenCV的索引是从0开始的。
阅读全文
相关推荐
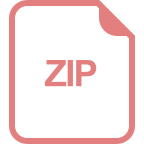
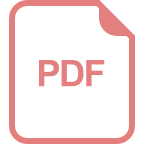

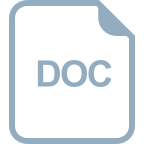
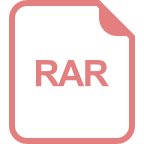
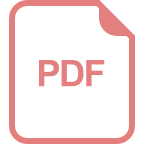
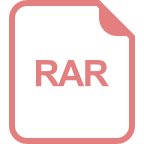
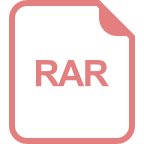
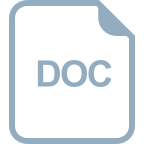
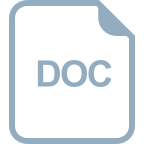
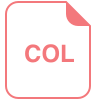
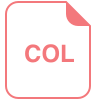
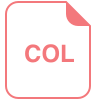




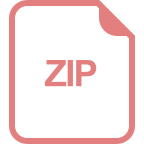