protobuf DebugString c++ 还原
时间: 2024-12-21 22:24:28 浏览: 9
在Protobuf (Protocol Buffers) 中,`DebugString()` 是一个函数用于将序列化后的消息转换成字符串形式,便于调试。在 C++ 中,当你有一个 `protobuf::Message` 或其派生类的对象,你可以通过这个方法获取一个包含原始数据和结构信息的调试字符串。
例如:
```cpp
#include <google/protobuf/message.h>
...
// 创建一个 Protobuf 消息实例
MyMessage message;
// 设置消息内容...
// 调用 DebugString() 函数
std::string debug_string = message.DebugString();
// 现在 debug_string 包含了原始消息的信息,可用于打印日志或调试
```
如果你想从这个调试字符串还原原始的消息,你需要解析这个字符串并构造一个新的 `MyMessage` 对象。但是,`DebugString()` 本身并不提供直接的数据反序列化功能,因为它的目的是展示消息的内部表示,而不是恢复原始数据。要从字符串还原,通常需要先解析字符串,然后使用 `ParseFromString()` 方法:
```cpp
// 假设你知道如何从 debug_string 分析出原始字节
std::vector<uint8_t> bytes(debug_string.begin(), debug_string.end());
message.ParseFromString(bytes);
```
相关问题
protobuf DebugString反解
Protocol Buffers(简称protobuf)是一种由Google开发的序列化数据语言,它将结构化的数据定义成.proto文件,然后可以生成多种语言的代码实现数据的编码和解码。DebugString是一个用于调试和打印的函数,它会返回一个字符串,展示了protobuf消息的内容。
当你想要查看protobuf消息在内存中的表示,可以使用`DebugString()`方法。这个方法通常在protobuf对象上调用,返回的是一个易于理解的文本格式,包含了字段名、类型和对应的值。例如:
```cpp
Message message;
// ...填充message内容
std::string debug_string = message.DebugString();
```
在Python中,也有类似的概念:
```python
from google.protobuf.json_format import MessageToJson
debug_str = message.SerializeToString()
# 或者直接转换为json字符串
json_str = MessageToJson(message)
```
然而,DebugString不是用来实际反序列化数据的,如果你想从这个字符串还原原始数据,你应该使用protobuf的解析功能,如`ParseFromString()`方法或`google.protobuf.json_format.Parse()`函数。
c++ protobuf 转string
在 C++ 中,将 protobuf 对象转换为字符串可以使用 protobuf 库中的 `SerializeToString()` 函数。该函数的原型如下:
```c++
bool SerializeToString(string* output) const;
```
其中,`output` 是一个指向 `string` 对象的指针,该函数将序列化后的数据写入 `output` 所指向的 `string` 对象中。
以下是一个示例代码,演示了如何将一个 protobuf 对象转换为字符串:
```c++
#include <iostream>
#include <string>
#include "my_proto.pb.h"
using namespace std;
int main()
{
// 创建一个 MyProto 对象
MyProto my_proto;
my_proto.set_id(123);
my_proto.set_name("test");
// 将 MyProto 对象序列化为字符串
string output;
if (my_proto.SerializeToString(&output))
{
// 输出序列化后的字符串
cout << "Serialized string: " << output << endl;
}
else
{
cout << "Failed to serialize MyProto object." << endl;
}
return 0;
}
```
在上面的示例中,我们首先创建了一个 `MyProto` 对象,并设置了它的 `id` 和 `name` 属性。然后,我们使用 `my_proto.SerializeToString(&output)` 函数将该对象序列化为字符串,并将结果写入 `output` 对象中。最后,我们输出了序列化后的字符串。
阅读全文
相关推荐
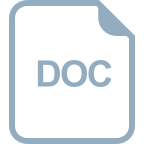
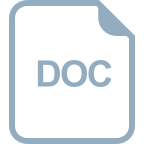
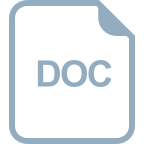
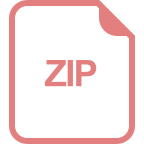
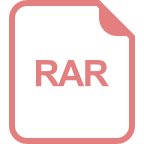
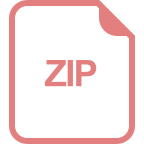
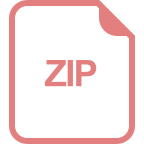
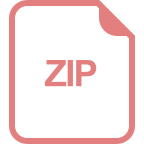
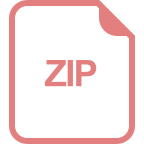
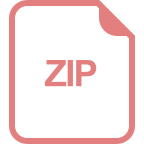




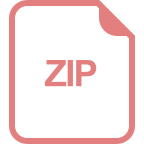
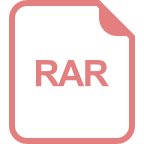