定义五个结构体数据类型student,包括姓名,年龄,性别,成绩,然后分别定义一个结构体变量和指针变量,分别实现对student的赋值并输出
时间: 2024-11-12 10:45:54 浏览: 155
在C语言中,你可以定义一个名为`Student`的结构体来包含学生的个人信息,如下所示:
```c
#include <stdio.h>
#include <string.h>
// 结构体定义
struct Student {
char name[50];
int age;
char gender; // 使用char存储性别,如'M'代表男性,'F'代表女性
float score;
};
int main() {
// 定义结构体变量
struct Student student1; // 声明一个结构体变量
// 赋值给学生1
strcpy(student1.name, "张三"); // 设置姓名
student1.age = 18; // 设置年龄
student1.gender = 'M'; // 设置性别
student1.score = 90.5f; // 设置成绩
// 输出学生信息
printf("Name: %s\n", student1.name);
printf("Age: %d\n", student1.age);
printf("Gender: %c\n", student1.gender);
printf("Score: %.1f\n", student1.score);
// 定义结构体指针
struct Student *ptrStudent; // 声明一个指向Student类型的指针
// 动态分配内存给指针
ptrStudent = (struct Student*)malloc(sizeof(struct Student));
// 如果内存分配成功
if (ptrStudent != NULL) {
// 给指针赋值,并同样设置信息
strcpy(ptrStudent->name, "李四");
ptrStudent->age = 20;
ptrStudent->gender = 'F';
ptrStudent->score = 87.0f;
// 输出指针指向的学生信息
printf("Name: %s\n", ptrStudent->name);
printf("Age: %d\n", ptrStudent->age);
printf("Gender: %c\n", ptrStudent->gender);
printf("Score: %.1f\n", ptrStudent->score);
// 释放内存
free(ptrStudent);
} else {
printf("Memory allocation failed.\n");
}
return 0;
}
```
阅读全文
相关推荐
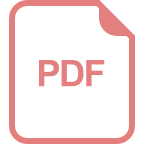
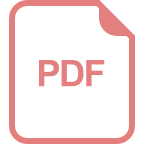
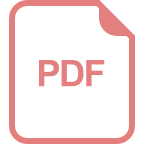















