python将16位深度图像转成8位
时间: 2024-05-03 18:22:04 浏览: 11
您好!对于您的问题,我很高兴为您提供帮助。
您可以使用以下代码将16位深度图像转换为8位:
```python
import cv2
import numpy as np
# 读入16位深度图像
depth_img = cv2.imread('depth_image_16bit.png', cv2.IMREAD_ANYDEPTH)
# 将像素值调整到0-255范围内
depth_scale = np.max(depth_img) / 255.0
depth_img_8bit = (depth_img / depth_scale).astype(np.uint8)
# 写出8位深度图像
cv2.imwrite('depth_image_8bit.png', depth_img_8bit)
```
以上代码会读入名为`depth_image_16bit.png`的16位深度图像,将其像素值调整到0-255范围内,并将其转换为8位深度图像,最后将转换后的8位深度图像写出到名为`depth_image_8bit.png`的文件中。
希望这个回答能够帮到您,如果您有其他问题,可以继续提出。
相关问题
python将8位深度图转点云示例
以下是一个示例代码,可以将8位深度图转换为点云:
```python
import numpy as np
# 读取深度图像
depth_image = cv2.imread('depth_image.png', cv2.IMREAD_UNCHANGED)
# 计算点云坐标
fx, fy, cx, cy = 600, 600, 320, 240 # 相机内参
rows, cols = depth_image.shape
u, v = np.meshgrid(range(cols), range(rows))
z = depth_image / 1000.0 # 将深度图像转换为深度值(单位:米)
x = np.multiply((u - cx) / fx, z)
y = np.multiply((v - cy) / fy, z)
cloud = np.stack((x, y, z), axis=-1)
# 保存点云数据
np.savetxt('point_cloud.txt', cloud.reshape((-1, 3)))
```
说明:
1. 读取深度图像时,我们需要使用 `cv2.IMREAD_UNCHANGED` 标志,使得深度图像能够以未修改的形式加载。
2. 计算点云坐标时,需要使用相机的内参(即相机的焦距和光心),以及深度图像中每个像素的坐标。
3. 为了将深度图像转换为真实深度值,我们将每个像素值除以1000。
4. 最后,我们将点云数据保存到文本文件中,每行包含一个点的X,Y,Z坐标。
python怎么将96位深度的图像改成为32位
可以使用Python的图像处理库Pillow来将96位深度的图像转换为32位深度的图像。具体步骤如下:
1. 安装Pillow库,可以在命令行中输入以下命令进行安装:
```
pip install Pillow
```
2. 使用Pillow库中的`Image`模块打开需要转换深度的图像文件,例如:
```
from PIL import Image
img = Image.open('example.png')
```
3. 将图像转换为32位深度,可以使用`Image.convert()`方法,并指定模式为“RGBA”:
```
img = img.convert('RGBA')
```
4. 最后保存转换后的图像,可以使用`Image.save()`方法:
```
img.save('example_32bit.png')
```
完整代码如下:
```
from PIL import Image
# 打开图像文件
img = Image.open('example.png')
# 将图像转换为32位深度
img = img.convert('RGBA')
# 保存转换后的图像
img.save('example_32bit.png')
```
请注意,转换后的图像可能会有一些色彩信息的丢失,因此在转换前建议备份原始图像文件。
相关推荐
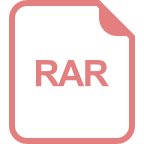
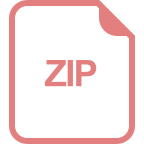












