c++ 用throw 抛字符串 如何定义字符串
时间: 2023-08-20 08:32:49 浏览: 68
在 C++ 中,可以使用字符串字面值或者 std::string 类型来定义字符串。在使用 throw 抛出异常时,需要将字符串转换为 const char* 类型。
以下是两种定义字符串的方法:
1.使用字符串字面值:
```c++
throw "Error: Something went wrong!";
```
2.使用 std::string 类型:
```c++
#include <string>
void foo() {
std::string errorMsg = "Error: Something went wrong!";
throw errorMsg.c_str();
}
```
需要注意的是,使用字符串字面值抛出异常时,需要确保该字符串在抛出异常时仍然有效,不会被销毁。因此,最好使用第二种方法,将字符串存储在 std::string 类型的变量中,再使用其 c_str() 方法将其转换为 const char* 类型。
相关问题
提供c++代码的opus解码字符串的代码
以下是使用 Opus 库在 C++ 中解码字符串的代码示例:
```c++
#include <opus/opus.h>
#include <string>
std::string decodeOpus(const uint8_t* compressedData, size_t dataSize) {
OpusDecoder* decoder = opus_decoder_create(48000, 2, nullptr);
if (!decoder) {
throw std::runtime_error("Failed to create Opus decoder");
}
const int OPUS_FRAME_SIZE = 960;
const int MAX_SAMPLES_PER_FRAME = OPUS_FRAME_SIZE * 2; // 2 channels
const int MAX_SAMPLES_PER_PACKET = MAX_SAMPLES_PER_FRAME * 60; // 60 frames per packet
const int MAX_OUTPUT_SAMPLES = MAX_SAMPLES_PER_PACKET * 2; // 2 channels
int outputBuffer[MAX_OUTPUT_SAMPLES];
int frameSize = opus_decoder_get_size(2);
if (frameSize < 0) {
opus_decoder_destroy(decoder);
throw std::runtime_error("Failed to get Opus decoder frame size");
}
int decodedSamples = opus_decode(decoder, compressedData, dataSize, outputBuffer, MAX_OUTPUT_SAMPLES, 0);
if (decodedSamples < 0) {
opus_decoder_destroy(decoder);
throw std::runtime_error("Failed to decode Opus data");
}
std::string decodedString(reinterpret_cast<const char*>(outputBuffer), decodedSamples * sizeof(int16_t));
opus_decoder_destroy(decoder);
return decodedString;
}
```
解码过程中的关键步骤:
1. 创建 Opus 解码器实例:`OpusDecoder* decoder = opus_decoder_create(48000, 2, nullptr);`
2. 定义解码缓冲区:`int outputBuffer[MAX_OUTPUT_SAMPLES];`
3. 调用 `opus_decode` 函数解码:`int decodedSamples = opus_decode(decoder, compressedData, dataSize, outputBuffer, MAX_OUTPUT_SAMPLES, 0);`
4. 将解码后的数据转换成字符串:`std::string decodedString(reinterpret_cast<const char*>(outputBuffer), decodedSamples * sizeof(int16_t));`
其中,`compressedData` 是包含 Opus 压缩数据的指针,`dataSize` 是数据大小。函数返回解码后的字符串。
c++ zlib 解压很大的gzip字符串
你可以使用Zlib库来解压gzip字符串。下面是一个简单的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <cstring>
#include <stdexcept>
#include <zlib.h>
std::string gzip_decompress(const std::string& compressed_string) {
const size_t BUFFER_SIZE = 1024 * 1024;
char buffer[BUFFER_SIZE];
std::stringstream uncompressed_stream;
z_stream zs;
memset(&zs, 0, sizeof(zs));
if (inflateInit2(&zs, 16 + MAX_WBITS) != Z_OK) {
throw std::runtime_error("inflateInit2 failed while decompressing.");
}
zs.next_in = (Bytef*)compressed_string.data();
zs.avail_in = compressed_string.size();
int ret;
do {
zs.next_out = reinterpret_cast<Bytef*>(buffer);
zs.avail_out = BUFFER_SIZE;
ret = inflate(&zs, 0);
if (uncompressed_stream.tellp() < 0) {
throw std::runtime_error("uncompressed stream error while decompressing.");
}
uncompressed_stream.write(buffer, BUFFER_SIZE - zs.avail_out);
} while (ret == Z_OK);
inflateEnd(&zs);
if (ret != Z_STREAM_END) {
throw std::runtime_error("corrupted compressed string while decompressing.");
}
return uncompressed_stream.str();
}
int main() {
std::ifstream input_file("compressed.gz", std::ios::in | std::ios::binary);
std::stringstream compressed_stream;
compressed_stream << input_file.rdbuf();
std::string compressed_string = compressed_stream.str();
std::string uncompressed_string = gzip_decompress(compressed_string);
std::cout << uncompressed_string << std::endl;
return 0;
}
```
在上面的代码中,我们定义了一个`gzip_decompress`函数,它接受一个压缩的字符串,并返回解压后的字符串。我们使用Zlib库的`inflate`函数来逐步解压字符串,并将解压后的数据写入到一个`std::stringstream`中。最后,我们将`std::stringstream`中的数据转换为一个字符串并返回它。
需要注意的一点是,我们使用了一个1MB大小的缓冲区来保存解压后的数据,这个缓冲区的大小可以根据你的实际需求进行调整。
相关推荐
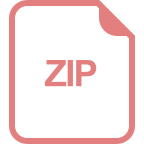
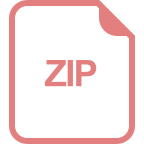
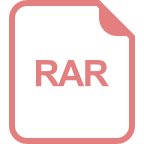
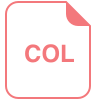











