举个更详细的例子
时间: 2023-10-20 22:07:16 浏览: 71
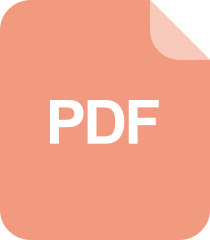
windowsAPI函数大全[归纳].pdf
好的,我们来举个更详细的例子。
假设我们要测试一个名为"my_math"的模块,其中包含一个名为"add"的函数,用于计算两个数的和。我们希望在测试函数中使用该函数,并且需要一个名为"test_data"的fixture,它会提供测试数据。
首先,我们需要在conftest.py文件中定义"test_data" fixture:
```python
import pytest
@pytest.fixture
def test_data():
return (1, 2, 3)
```
这个fixture返回一个元组,其中包含三个数1、2、3。接下来,我们需要在测试函数中使用该fixture和"add"函数:
```python
from my_math import add
def test_add(test_data):
x, y, expected_result = test_data
result = add(x, y)
assert result == expected_result
```
这个测试函数使用了"test_data" fixture,它会返回一个元组,其中包含三个数1、2、3。接着,我们使用元组的解包方式,将1和2分别赋值给x和y变量,将3赋值给expected_result变量。然后,我们调用"add"函数计算x和y的和,将结果赋值给result变量。最后,我们使用assert语句检查result是否等于expected_result。
但是,如果我们在测试函数中使用了一个名为"db"的fixture,它会返回一个连接到数据库的对象,我们需要确保"db" fixture在"test_data" fixture之前执行,从而保证测试数据已经准备好了。我们可以使用pytest的dependency机制来实现这个目的:
```python
import pytest
from my_math import add
@pytest.fixture
def test_data():
return (1, 2, 3)
@pytest.fixture
def db():
# 连接到数据库
return db_connection
@pytest.fixture(dependencies=["test_data"])
def add_fixture(test_data):
x, y, expected_result = test_data
result = add(x, y)
return (result, expected_result)
def test_add(add_fixture, db):
result, expected_result = add_fixture
# 使用result和expected_result进行测试
```
在这个例子中,我们定义了一个名为"db"的fixture,它会返回一个连接到数据库的对象。同时,我们定义了一个名为"add_fixture"的fixture,它依赖于"test_data" fixture,并且返回一个元组,其中包含"add"函数的计算结果和预期结果。我们在测试函数中使用了"add_fixture"和"db" fixture。pytest会先执行"test_data" fixture,然后执行"add_fixture" fixture,最后执行"db" fixture,从而保证测试数据已经准备好了。
阅读全文
相关推荐
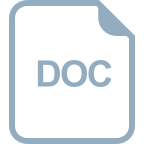
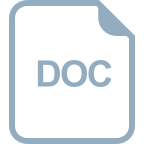















