python图像旋转数学公式
时间: 2023-06-27 13:08:13 浏览: 68
假设要将一个点 $(x,y)$ 绕原点逆时针旋转角度 $\theta$,则旋转后的点坐标为 $(x',y')$,其数学公式为:
$$
\begin{aligned}
x' &= x\cos\theta - y\sin\theta \\
y' &= x\sin\theta + y\cos\theta
\end{aligned}
$$
如果要将整个图像逆时针旋转角度 $\theta$,则可以对每个像素点 $(x,y)$ 应用上述公式。在 Python 中,可以使用 NumPy 库提供的矩阵变换函数 `np.dot` 来实现:
```python
import numpy as np
def rotate_image(image, angle):
"""
将图像逆时针旋转给定角度(单位:度)
"""
# 以图像中心为旋转中心
cx, cy = image.shape[1] // 2, image.shape[0] // 2
# 计算旋转矩阵
theta = np.deg2rad(angle)
c, s = np.cos(theta), np.sin(theta)
rotation_matrix = np.array([[c, -s], [s, c]])
# 对每个像素点应用旋转矩阵
height, width = image.shape[:2]
x, y = np.meshgrid(np.arange(width), np.arange(height))
x, y = x - cx, y - cy
xy = np.stack([x, y], axis=-1)
xy_rotated = np.dot(xy, rotation_matrix.T)
x_rotated, y_rotated = xy_rotated[..., 0], xy_rotated[..., 1]
x_rotated, y_rotated = x_rotated + cx, y_rotated + cy
# 对旋转后的坐标进行插值,得到旋转后的图像
rotated_image = cv2.remap(image, x_rotated.astype(np.float32), y_rotated.astype(np.float32), cv2.INTER_LINEAR)
return rotated_image
```
其中,`image` 是要旋转的图像,`angle` 是旋转角度。函数首先计算旋转中心(即图像中心),然后计算旋转矩阵,最后对每个像素点应用旋转矩阵,并使用 OpenCV 提供的插值函数 `cv2.remap` 进行坐标插值,得到旋转后的图像。
相关推荐
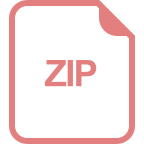
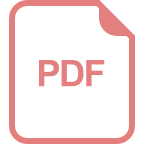














