数据结构哈希查找C语言
时间: 2023-07-22 18:09:17 浏览: 139
哈希查找是一种通过哈希函数将关键字映射到哈希表中的位置,然后在该位置进行查找的方法。在C语言中,我们可以使用数组来实现哈希表。
首先,需要定义一个哈希表的结构体,包含两个成员:数组和数组长度。数组的每个元素是一个链表的头节点,用于解决哈希冲突。
```c
#define MAX_SIZE 100
// 链表节点
typedef struct ListNode {
int key;
int value;
struct ListNode* next;
} ListNode;
// 哈希表
typedef struct HashTable {
ListNode* array[MAX_SIZE];
int size;
} HashTable;
```
然后,需要实现以下几个操作:
1. 哈希函数:将关键字映射为哈希表的位置。
```c
int hashFunction(int key, int size) {
return key % size;
}
```
2. 初始化哈希表。
```c
void initHashTable(HashTable* hashTable, int size) {
hashTable->size = size;
for (int i = 0; i < size; i++) {
hashTable->array[i] = NULL;
}
}
```
3. 插入元素。
```c
void insert(HashTable* hashTable, int key, int value) {
int index = hashFunction(key, hashTable->size);
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->key = key;
newNode->value = value;
newNode->next = NULL;
if (hashTable->array[index] == NULL) {
hashTable->array[index] = newNode;
} else {
ListNode* cur = hashTable->array[index];
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
}
```
4. 查找元素。
```c
int search(HashTable* hashTable, int key) {
int index = hashFunction(key, hashTable->size);
ListNode* cur = hashTable->array[index];
while (cur != NULL) {
if (cur->key == key) {
return cur->value;
}
cur = cur->next;
}
return -1; // 没有找到
}
```
这样,我们就可以使用哈希查找来快速检索数据了。注意,在实际应用中,还需要处理哈希冲突的情况,例如使用链地址法解决冲突。另外,还需要实现删除操作等其他功能。以上只是一个简单的哈希查找的示例。
阅读全文
相关推荐
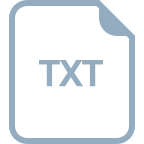
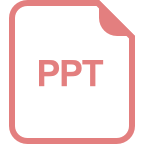
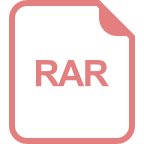
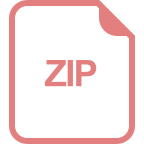
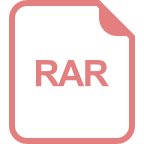
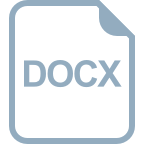
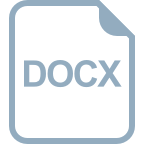
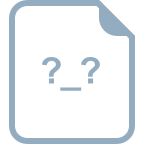
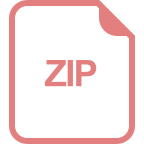
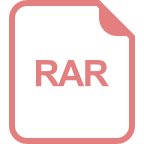
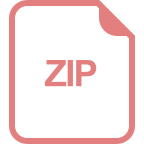
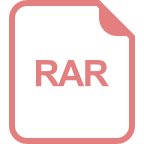
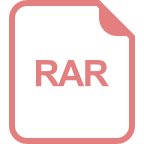