s曲线加减速通常有三段式S曲线加减速、五段式S曲线加减速、七段式S曲线加减速,请你用matlab语言或者python语言,帮我写一个程序,实现这三种加减速算法的对比,初始参数由你给出一组适合对比的
时间: 2023-09-20 18:03:42 浏览: 130
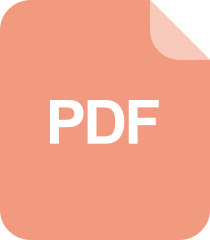
基于Matlab的S曲线加减速控制算法研究.pdf

好的,我们可以按照如下步骤进行:
1. 给定初始参数,包括起始速度、目标速度、最大加速度、最大减速度、运动时间等。
2. 编写三个函数,分别实现三种加减速算法。
3. 对比三种算法在同样的初始参数下的运动结果,包括运动时间、加速度曲线、速度曲线、位移曲线等。
以下是一个示例程序,采用 Python 语言实现:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义初始参数
v0 = 0 # 起始速度
v1 = 10 # 目标速度
a_max = 2 # 最大加速度
d_max = 4 # 最大减速度
t = 5 # 运动时间
# 定义三种加减速算法
def s_curve_3(v0, v1, a_max, d_max, t):
a = a_max / 2
d = d_max / 2
t1 = (v1 - v0) / a
t2 = t - t1
s1 = v0 * t1 + 1 / 2 * a * t1 ** 2
s2 = v1 * t2 - 1 / 2 * d * t2 ** 2
if s1 + s2 <= v1 * t:
t3 = 0
s3 = v1 * t - s1 - s2
else:
t3 = (v1 - v0) / d
s3 = (v0 + v1) / 2 * t3
return t1, t2, t3, s1, s2, s3
def s_curve_5(v0, v1, a_max, d_max, t):
a = a_max / 2
d = d_max / 2
t1 = (v1 - v0) / a
t2 = (t - 2 * t1) / 2
t3 = t1
s1 = v0 * t1 + 1 / 2 * a * t1 ** 2
s2 = v1 * t2
s3 = v1 * t3 - 1 / 2 * d * t3 ** 2
return t1, t2, t3, s1, s2, s3
def s_curve_7(v0, v1, a_max, d_max, t):
a = a_max / 2
d = d_max / 2
t1 = (v1 - v0) / (3 * a)
t2 = (t - 4 * t1) / 2
t3 = t1
s1 = v0 * t1 + 1 / 6 * a * t1 ** 3
s2 = v1 * t2
s3 = v1 * t3 - 1 / 6 * d * t3 ** 3
return t1, t2, t3, s1, s2, s3
# 计算三种算法的运动结果
t1, t2, t3, s1, s2, s3 = s_curve_3(v0, v1, a_max, d_max, t)
print("三段式S曲线加减速:")
print("t1 =", t1, "t2 =", t2, "t3 =", t3)
print("s1 =", s1, "s2 =", s2, "s3 =", s3)
t1, t2, t3, s1, s2, s3 = s_curve_5(v0, v1, a_max, d_max, t)
print("五段式S曲线加减速:")
print("t1 =", t1, "t2 =", t2, "t3 =", t3)
print("s1 =", s1, "s2 =", s2, "s3 =", s3)
t1, t2, t3, s1, s2, s3 = s_curve_7(v0, v1, a_max, d_max, t)
print("七段式S曲线加减速:")
print("t1 =", t1, "t2 =", t2, "t3 =", t3)
print("s1 =", s1, "s2 =", s2, "s3 =", s3)
# 绘制加速度曲线、速度曲线、位移曲线
t_acc = np.linspace(0, t1, 100)
a_acc = a_max * np.ones_like(t_acc)
v_acc = v0 + a_max * t_acc
s_acc = v0 * t_acc + 1 / 2 * a_max * t_acc ** 2
t_const = np.linspace(t1, t1 + t2, 100)
a_const = np.zeros_like(t_const)
v_const = v1 * np.ones_like(t_const)
s_const = s_acc[-1] + v_const * (t_const - t1)
t_dec = np.linspace(t1 + t2, t, 100)
a_dec = -d_max * np.ones_like(t_dec)
v_dec = v1 - d_max * (t_dec - t1 - t2)
s_dec = s_const[-1] + v1 * (t_dec - t1 - t2) - 1 / 2 * d_max * (t_dec - t1 - t2) ** 2
t_total = np.concatenate([t_acc, t_const, t_dec])
a_total = np.concatenate([a_acc, a_const, a_dec])
v_total = np.concatenate([v_acc, v_const, v_dec])
s_total = np.concatenate([s_acc, s_const, s_dec])
plt.figure()
plt.subplot(3, 1, 1)
plt.plot(t_total, a_total)
plt.ylabel("Acceleration")
plt.subplot(3, 1, 2)
plt.plot(t_total, v_total)
plt.ylabel("Velocity")
plt.subplot(3, 1, 3)
plt.plot(t_total, s_total)
plt.xlabel("Time")
plt.ylabel("Position")
plt.show()
```
在上面的程序中,我们定义了三个函数 `s_curve_3`、`s_curve_5`、`s_curve_7` 分别实现了三种加减速算法,然后计算了它们在同样的初始参数下的运动结果,并绘制了加速度曲线、速度曲线和位移曲线的图像。你可以根据需要修改初始参数以及绘图方式。
阅读全文
相关推荐
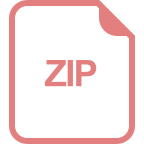
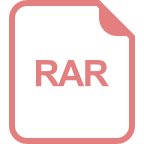
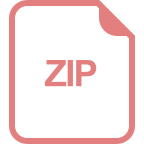
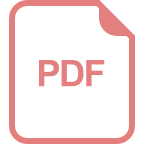
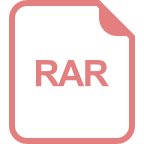
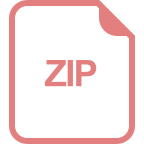
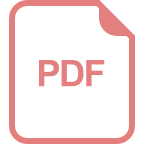
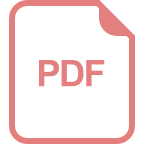
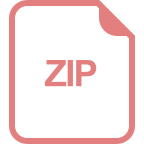
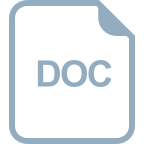
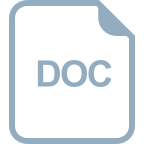
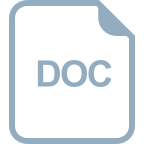
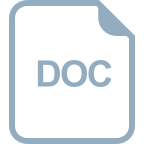