ShellExecute(NULL,_T("OPEN"),_T("C:\\Users\\CTOS.CTOS-PC\\Desktop\\DEXSorter1.1 基础版1\\DEXSorter\\bmp\\"),NULL,NULL,SW_SHOW);
时间: 2024-04-19 18:22:25 浏览: 91
ShellExecute是Windows API中的一个函数,用于执行外部程序或打开文件。它的参数包括:
1. 第一个参数是一个窗口句柄,通常传入NULL表示使用当前活动窗口。
2. 第二个参数是一个字符串,指定要执行的操作,比如"OPEN"表示打开文件。
3. 第三个参数是一个字符串,指定要执行的文件路径或者命令。
4. 第四个参数是一个字符串,指定命令行参数,可以为空。
5. 第五个参数是一个字符串,指定工作目录,可以为空。
6. 第六个参数是一个整数,指定窗口的显示方式。
所以,ShellExecute(NULL,_T("OPEN"),_T("C:\\Users\\CTOS.CTOS-PC\\Desktop\\DEXSorter1.1 基础版1\\DEXSorter\\bmp\\"),NULL,NULL,SW_SHOW)的作用是打开指定路径下的文件夹。
相关问题
const TCHAR szOperation[] = _T("open"); const TCHAR szAddress[] = _T("http://www.ssoier.cn:8088/ocr/index.php"); int WINAPI WinMain(HINSTANCE hInst, HINSTANCE, LPSTR lpCmd, int nShow); { HINSTANCE hRslt = ShellExecute(NULL, szOperation, szAddress, NULL, NULL, SW_SHOWNORMAL); assert(hRslt > (HINSTANCE)HINSTANCE_ERROR); exit(100); }
这段代码是一个简单的C++程序,它使用了Windows API函数`ShellExecute`来打开一个指定网址的默认浏览器。在`WinMain`函数中,我们调用`ShellExecute`函数,并传递了打开操作、网址以及其他参数。然后使用`assert`断言来检查函数调用是否成功,最后使用`exit`函数退出程序。
需要注意的是,这段代码并不与题目要求的数学表达式输入内容相关。如果你想要实现自动输入数学表达式并计算结果,可以使用C++的输入输出流和适当的算法来解析和计算表达式。以下是一个示例代码来实现这个功能:
```cpp
#include <iostream>
#include <string>
#include <stack>
using namespace std;
int calculateExpression(const string& expression) {
stack<int> numbers;
stack<char> operators;
for (int i = 0; i < expression.length(); i++) {
if (expression[i] >= '0' && expression[i] <= '9') {
int num = 0;
while (i < expression.length() && expression[i] >= '0' && expression[i] <= '9') {
num = num * 10 + (expression[i] - '0');
i++;
}
i--;
numbers.push(num);
}
else if (expression[i] == '+' || expression[i] == '-') {
while (!operators.empty() && (operators.top() == '+' || operators.top() == '-' ||
operators.top() == '*' || operators.top() == '/')) {
int b = numbers.top();
numbers.pop();
int a = numbers.top();
numbers.pop();
char op = operators.top();
operators.pop();
if (op == '+') {
numbers.push(a + b);
}
else if (op == '-') {
numbers.push(a - b);
}
else if (op == '*') {
numbers.push(a * b);
}
else if (op == '/') {
numbers.push(a / b);
}
}
operators.push(expression[i]);
}
else if (expression[i] == '*' || expression[i] == '/') {
while (!operators.empty() && (operators.top() == '*' || operators.top() == '/')) {
int b = numbers.top();
numbers.pop();
int a = numbers.top();
numbers.pop();
char op = operators.top();
operators.pop();
if (op == '*') {
numbers.push(a * b);
}
else if (op == '/') {
numbers.push(a / b);
}
}
operators.push(expression[i]);
}
}
while (!operators.empty()) {
int b = numbers.top();
numbers.pop();
int a = numbers.top();
numbers.pop();
char op = operators.top();
operators.pop();
if (op == '+') {
numbers.push(a + b);
}
else if (op == '-') {
numbers.push(a - b);
}
else if (op == '*') {
numbers.push(a * b);
}
else if (op == '/') {
numbers.push(a / b);
}
}
return numbers.top();
}
int main() {
string expression;
cout << "请输入数学表达式: ";
cin >> expression;
int result = calculateExpression(expression);
cout << "结果为: " << result << endl;
return 0;
}
```
这个示例代码会提示用户输入一个数学表达式,然后使用`calculateExpression`函数计算表达式的结果,并输出结果。你可以根据你的具体需求进行修改和扩展。
#define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdlib.h> #include <Windows.h> #include <string.h> #include <direct.h> #include<malloc.h> int main(int argc, char* argv[]) { char* path; char OpenFile[1000]; int k = 0; path = (char*)malloc(1000); _getcwd(path, 1000); int i; for (i = strlen(argv[1]) - 1; i >= 0; i--) { if (argv[1][i] == '\\' || argv[1][i] == '/') { k++; break; } } strcat(path, "\\AnswerH_5"); if (k == 0) strcat(path, argv[1]); else strcat(path, argv[1] + i); for (i = strlen(path) - 1;i >= 0;i--) { if (path[i] == '.') { path[i] = 0; break; } } strcat(path, "H.html"); int w_num, ch_num, num_num, bl_num, let_num, o_num; FILE* txt_fp; if ((txt_fp = fopen(argv[1], "r")) == NULL) exit(0); fscanf(txt_fp, "The number of words:%d\n", &w_num); fscanf(txt_fp, "The number of characters:%d\n", &ch_num); fscanf(txt_fp, "The number of numbers:%d\n", &num_num); fscanf(txt_fp, "The number of blanks:%d\n", &bl_num); fscanf(txt_fp, "The number of letters:%d\n", &let_num); fscanf(txt_fp, "The number of others:%d\n", &o_num); fclose(txt_fp); //扫描txt文件 FILE* html_fp; if ((html_fp = fopen(path, "w+")) == NULL) exit(0); fprintf(html_fp, "The number of words:%d\n", w_num); fprintf(html_fp, "The number of characters:%d\n", ch_num); fprintf(html_fp, "The number of numbers:%d\n", num_num); fprintf(html_fp, "The number of blanks:%d\n", bl_num); fprintf(html_fp, "The number of letters:%d\n", let_num); fprintf(html_fp, "The number of others:%d\n", o_num); fclose(html_fp); //编写html文件 if (strcmp(argv[2], "auto") == 0) { strcpy(OpenFile, "C:\\Program Files\\Internet Explorer\\IEXPLORE.EXE "); strcat(OpenFile, path); WinExec(OpenFile, SW_SHOWMAXIMIZED); //ShellExecute(0,"open",path, 0,0,1);// } if (strcmp(argv[2], "quiet") == 0) { printf("已生成html文件,文件路径为:%s", path); } }
这段代码是一个文件读取程序,它可以读取一个文本文件,并将文件中的单词数、字符数、数字数、空格数、字母数和其他字符数统计出来,然后将这些统计结果写入一个 HTML 文件中。代码使用了命令行参数,第一个参数是要读取的文本文件的路径,第二个参数是程序运行方式,可以是 "auto" 或 "quiet"。如果是 "auto",程序会在生成 HTML 文件后自动打开它;如果是 "quiet",程序只会输出生成的 HTML 文件路径,不会打开文件。
具体实现过程是先使用 _getcwd 函数获取程序运行时所在的目录,然后根据命令行参数中指定的文件路径来拼接出 HTML 文件的路径。接着使用 fopen 函数打开文本文件,使用 fscanf 函数读取文件中的统计数据。然后使用 fprintf 函数将统计数据写入 HTML 文件中。最后根据命令行参数中指定的程序运行方式来打开 HTML 文件或者输出文件路径。
需要注意的是,这段代码使用了动态内存分配函数 malloc,因此需要在程序结束时使用 free 函数释放分配的内存,否则会导致内存泄漏。
阅读全文
相关推荐
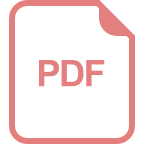
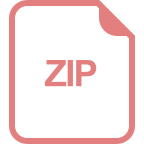
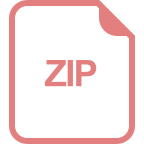
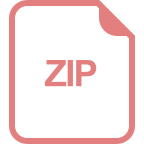
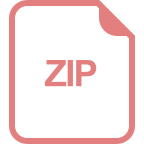
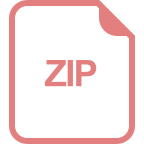
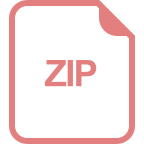
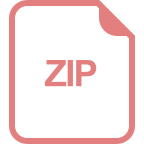
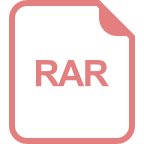
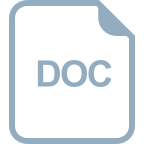





