Android 连接蓝牙
时间: 2023-07-28 19:07:36 浏览: 47
要在 Android 上连接蓝牙设备,您需要使用 BluetoothAdapter 和 BluetoothDevice 类。以下是一些连接蓝牙设备的基本步骤:
1. 获取 BluetoothAdapter 实例。您可以使用 getDefaultAdapter() 方法来获取默认的 BluetoothAdapter 实例:
```java
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
```
2. 检查设备是否支持蓝牙。可以使用 isEnabled() 方法检查设备是否已启用蓝牙:
```java
if (bluetoothAdapter == null || !bluetoothAdapter.isEnabled()) {
// 设备不支持蓝牙或蓝牙未启用
}
```
3. 扫描蓝牙设备并获取设备列表。可以使用 startDiscovery() 方法扫描蓝牙设备,使用 BroadcastReceiver 监听扫描结果,并使用 BluetoothDevice 类获取设备列表:
```java
bluetoothAdapter.startDiscovery();
private final BroadcastReceiver receiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
// 处理设备列表
}
}
};
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
registerReceiver(receiver, filter);
```
4. 连接蓝牙设备。可以使用 connectGatt() 方法连接蓝牙设备,并使用 BluetoothGattCallback 监听连接状态和数据传输:
```java
BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 设备已连接
gatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 设备已断开连接
}
}
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
// 处理服务发现
}
public void onCharacteristicRead(BluetoothGatt gatt,
BluetoothGattCharacteristic characteristic,
int status) {
// 处理特征值读取
}
public void onCharacteristicWrite(BluetoothGatt gatt,
BluetoothGattCharacteristic characteristic,
int status) {
// 处理特征值写入
}
// 其他回调方法
};
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
BluetoothGatt gatt = device.connectGatt(context, false, gattCallback);
```
这些是连接蓝牙设备的基本步骤,您可以根据需要进行调整和扩展。请注意,不同的蓝牙设备可能需要不同的连接方式和协议,您需要根据设备文档或开发人员指南进行相应的设置和操作。
相关推荐
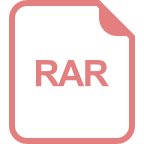














