rpc Demo with rust
时间: 2024-02-02 18:41:32 浏览: 28
Sure, I can help you with that! Rust has a built-in library for handling RPC (remote procedure call) communication called `tonic`. You can use this library to create both server and client components for your RPC interface. Here's a basic example of how to create a simple RPC demo:
1. Define your service interface using Protocol Buffers (protobuf) IDL.
```protobuf
// example.proto
syntax = "proto3";
package example;
service Greeter {
rpc SayHello(HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
```
2. Use `tonic-build` to generate Rust code from your protobuf IDL.
```bash
$ cargo install protobuf-codegen
$ cargo install tonic-build
$ tonic-build example.proto
```
3. Implement your service using Rust.
```rust
// server.rs
use example::{greeter_server::Greeter, HelloRequest, HelloResponse};
use tonic::{transport::Server, Request, Response, Status};
#[derive(Default)]
pub struct GreeterService {}
#[tonic::async_trait]
impl Greeter for GreeterService {
async fn say_hello(&self, request: Request<HelloRequest>) -> Result<Response<HelloResponse>, Status> {
let name = &request.get_ref().name;
let message = format!("Hello, {}!", name);
let response = HelloResponse { message };
Ok(Response::new(response))
}
}
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let addr = "127.0.0.1:50051".parse()?;
let greeter_service = GreeterService::default();
println!("Listening on: {}", addr);
Server::builder()
.add_service(GreeterServer::new(greeter_service))
.serve(addr)
.await?;
Ok(())
}
```
4. Create a client for your service.
```rust
// client.rs
use example::{greeter_client::GreeterClient, HelloRequest};
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let addr = "http://127.0.0.1:50051";
let mut client = GreeterClient::connect(addr).await?;
let request = HelloRequest { name: "World".to_string() };
let response = client.say_hello(request).await?;
println!("Got response: {:?}", response);
Ok(())
}
```
5. Build and run both the server and client.
```bash
$ cargo build && ./target/debug/server
$ cargo build && ./target/debug/client
```
You should see output from the server saying it's listening on `127.0.0.1:50051` and output from the client saying it got a response with the message `"Hello, World!"`.
相关推荐
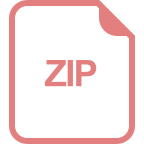
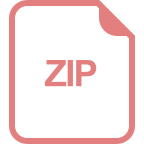














