多表联查,获取另外一张表的字段
时间: 2023-11-21 18:29:30 浏览: 114
多表联查是指在SQL语句中同时查询多张表的数据,并将它们关联起来。通过多表联查可以获取另外一张表的字段,具体的操作步骤如下:
1. 使用SELECT语句查询需要的字段,例如:
SELECT table1.field1, table2.field2
2. 使用FROM语句指定需要查询的表,例如:
FROM table1, table2
3. 使用JOIN语句将需要查询的表关联起来,例如:
JOIN table2 ON table1.key = table2.key
4. 最后使用WHERE语句限制查询条件,例如:
WHERE table1.field1 = 'value'
完整的SQL语句如下:
SELECT table1.field1, table2.field2
FROM table1
JOIN table2 ON table1.key = table2.key
WHERE table1.field1 = 'value'
相关问题
多表联查,获取另外一张表的字段 用java
可以使用Java中的JDBC(Java Database Connectivity)来执行多表联查。
以下是使用JDBC获取两张表的字段的示例代码:
```java
import java.sql.*;
public class MultiTableQueryExample {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// Register JDBC driver
Class.forName("com.mysql.jdbc.Driver");
// Open a connection
System.out.println("Connecting to database...");
conn = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password");
// Execute a query
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql = "SELECT orders.order_id, customers.customer_name, orders.order_date " +
"FROM orders " +
"INNER JOIN customers ON orders.customer_id=customers.customer_id";
rs = stmt.executeQuery(sql);
// Extract data from result set
while(rs.next()) {
// Retrieve by column name
int orderId = rs.getInt("order_id");
String customerName = rs.getString("customer_name");
Date orderDate = rs.getDate("order_date");
// Display values
System.out.print("Order ID: " + orderId);
System.out.print(", Customer Name: " + customerName);
System.out.println(", Order Date: " + orderDate);
}
} catch(SQLException se) {
// Handle errors for JDBC
se.printStackTrace();
} catch(Exception e) {
// Handle errors for Class.forName
e.printStackTrace();
} finally {
// Close resources
try {
if(rs != null) rs.close();
} catch(SQLException se) {
se.printStackTrace();
}
try {
if(stmt != null) stmt.close();
} catch(SQLException se) {
se.printStackTrace();
}
try {
if(conn != null) conn.close();
} catch(SQLException se) {
se.printStackTrace();
}
}
}
}
```
在上面的示例代码中,我们使用了一个INNER JOIN来连接两张表(orders和customers)并检索每个订单的订单ID,客户名称和订单日期。我们通过在SELECT语句中指定所需的列来选择要检索的数据。在while循环中,我们使用rs.getInt(),rs.getString()和rs.getDate()方法来检索结果集中的数据。最后,我们释放所有资源并关闭连接。
DB2实现联查两张表,其中一张表的字段是根据另一张表的内容确定的,不同内容获取字段不同
在DB2中,如果需要根据另一张表的内容动态查询并获取字段,可以使用`CASE`语句或者嵌套的`SELECT`语句结合`JOIN`来实现条件式字段选择。假设我们有两张表,Table1(主表)包含固定字段,而Table2(依赖表)有一些特定的分类信息对应不同的字段。以下是基本步骤:
1. 使用`JOIN`将Table1和Table2连接起来,通常使用`INNER JOIN`或`LEFT JOIN`,取决于是否所有Table1的记录都需要匹配Table2的信息。
```sql
SELECT Table1.Field1,
Table1.Field2,
CASE WHEN Table2.Category = 'CategoryA'
THEN Table2.FieldA
ELSE Table2.FieldB
END AS DynamicField
FROM Table1
LEFT JOIN Table2 ON Table1.Id = Table2.Table1Id;
```
2. `CASE`语句根据Table2的Category字段的值,动态选择返回Table2的不同字段。
如果你想根据更复杂的条件来选择字段,还可以增加更多的`WHEN`子句。
注意,这种做法适合数据结构相对稳定的情况,如果Table2的分类规则频繁变动,可能会增加维护复杂度。此外,如果需要处理大量的类别和对应的字段,考虑设计成视图或者存储过程会更为高效。
阅读全文
相关推荐



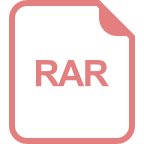





