如何用canvas实现一张图片绕尺寸为800*700居中的画布的中心点自动旋转
时间: 2024-02-05 15:14:07 浏览: 182
可以使用以下步骤来实现:
1. 获取画布和图片的元素,并计算出图片的中心点坐标。
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const img = document.getElementById('img');
const canvasWidth = 800;
const canvasHeight = 700;
const imgWidth = img.width;
const imgHeight = img.height;
const imgX = canvasWidth / 2;
const imgY = canvasHeight / 2;
```
2. 设置画布的宽度和高度,并将图片绘制在画布的中心点。
```javascript
canvas.width = canvasWidth;
canvas.height = canvasHeight;
ctx.translate(imgX, imgY);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
```
3. 使用`requestAnimationFrame`函数来更新图片的旋转角度,并将旋转后的图片绘制到画布上。
```javascript
let angle = 0;
function draw() {
angle += 0.01;
ctx.clearRect(-canvasWidth / 2, -canvasHeight / 2, canvasWidth, canvasHeight);
ctx.rotate(angle);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
requestAnimationFrame(draw);
}
draw();
```
完整的代码如下:
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const img = document.getElementById('img');
const canvasWidth = 800;
const canvasHeight = 700;
const imgWidth = img.width;
const imgHeight = img.height;
const imgX = canvasWidth / 2;
const imgY = canvasHeight / 2;
canvas.width = canvasWidth;
canvas.height = canvasHeight;
ctx.translate(imgX, imgY);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
let angle = 0;
function draw() {
angle += 0.01;
ctx.clearRect(-canvasWidth / 2, -canvasHeight / 2, canvasWidth, canvasHeight);
ctx.rotate(angle);
ctx.drawImage(img, -imgWidth / 2, -imgHeight / 2, imgWidth, imgHeight);
requestAnimationFrame(draw);
}
draw();
```
阅读全文
相关推荐

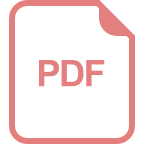
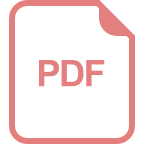
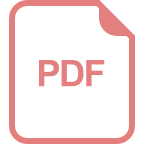
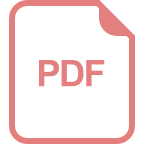
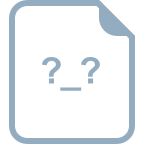
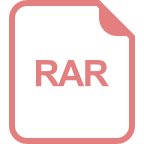
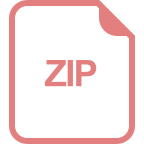
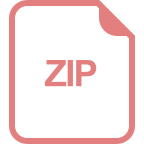
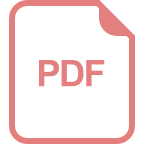
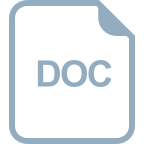
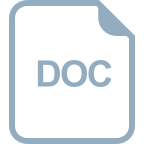
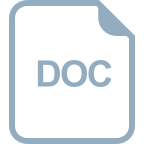
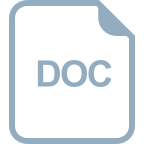
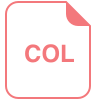
