python编写连数据库的点菜程序窗口
时间: 2023-08-10 17:03:44 浏览: 104
好的,下面是一个简单的Python编写带数据库连接的点菜程序窗口的示例代码:
```python
import tkinter as tk
import sqlite3
# 连接数据库
conn = sqlite3.connect("menu.db")
cur = conn.cursor()
# 创建菜单表
cur.execute("CREATE TABLE IF NOT EXISTS menu (id INTEGER PRIMARY KEY AUTOINCREMENT, category TEXT, name TEXT, price INTEGER)")
# 插入菜品数据
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("热菜", "宫保鸡丁", 25))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("热菜", "水煮肉片", 30))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("热菜", "鱼香肉丝", 20))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("凉菜", "拍黄瓜", 8))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("凉菜", "凉拌豆腐", 10))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("凉菜", "蒜泥白肉", 15))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("饮料", "可乐", 5))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("饮料", "雪碧", 5))
cur.execute("INSERT INTO menu (category, name, price) VALUES (?, ?, ?)", ("饮料", "矿泉水", 2))
conn.commit()
class App:
def __init__(self, master):
self.master = master
master.title("点菜程序")
# 创建菜单列表
self.menu_list = tk.Listbox(master, width=30)
self.menu_list.grid(row=0, column=0, rowspan=4)
self.menu_list.bind("<<ListboxSelect>>", self.show_price)
# 添加菜品到菜单列表
cur.execute("SELECT category, name, price FROM menu")
self.menu_items = []
for row in cur.fetchall():
self.menu_items.append(row)
self.menu_list.insert(tk.END, f"{row[0]}-{row[1]}")
# 创建菜品价格标签
self.price_label = tk.Label(master, text="价格:")
self.price_label.grid(row=0, column=1)
# 创建数量选择框和加入购物车按钮
self.quantity_var = tk.StringVar()
tk.Label(master, text="数量:").grid(row=1, column=1)
self.quantity_entry = tk.Entry(master, textvariable=self.quantity_var)
self.quantity_entry.grid(row=1, column=2)
tk.Button(master, text="加入购物车", command=self.add_to_cart).grid(row=2, column=1, columnspan=2)
# 创建购物车列表和结算按钮
self.cart_list = tk.Listbox(master, width=30)
self.cart_list.grid(row=3, column=0, rowspan=4)
tk.Button(master, text="结算", command=self.checkout).grid(row=7, column=0)
# 初始化购物车和总价
self.cart_items = {}
self.total_price = 0
def show_price(self, event):
# 显示所选菜品的价格
selection = self.menu_list.curselection()
if selection:
index = selection[0]
category, name, price = self.menu_items[index]
self.price_label.config(text=f"价格:{price}元")
def add_to_cart(self):
# 将所选菜品加入购物车
selection = self.menu_list.curselection()
if selection:
index = selection[0]
category, name, price = self.menu_items[index]
quantity = int(self.quantity_var.get())
if name in self.cart_items:
self.cart_items[name]["quantity"] += quantity
else:
self.cart_items[name] = {"price": price, "quantity": quantity}
self.total_price += price * quantity
self.update_cart_list()
def update_cart_list(self):
# 更新购物车列表
self.cart_list.delete(0, tk.END)
for name, info in self.cart_items.items():
price = info["price"]
quantity = info["quantity"]
self.cart_list.insert(tk.END, f"{name} {price}元 x {quantity}")
def checkout(self):
# 结算,并清空购物车和总价
tk.messagebox.showinfo("结算", f"总价格为:{self.total_price}元")
self.cart_items = {}
self.total_price = 0
self.update_cart_list()
root = tk.Tk()
app = App(root)
root.mainloop()
# 关闭数据库连接
conn.close()
```
这个程序使用了Tkinter模块创建了一个窗口,其中包含一个菜单列表、一个菜品价格标签、一个数量选择框和加入购物车按钮、一个购物车列表和结算按钮。程序会连接一个SQLite数据库,将菜单数据插入到menu表中,并从中读取数据来展示菜单列表。用户在菜单列表中选择菜品后,程序会显示该菜品的价格,并将其加入购物车。用户可以在数量选择框中输入所选菜品的数量,点击加入购物车按钮后,程序会将所选菜品及其数量加入购物车列表,并更新总价。当用户点击结算按钮时,程序会弹出一个消息框,显示总价,并清空购物车和总价。
阅读全文
相关推荐
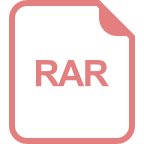
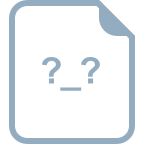
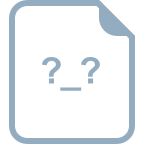
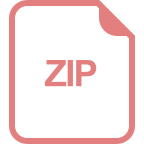
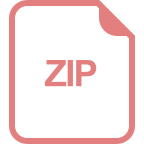
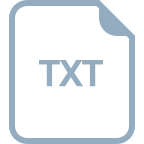
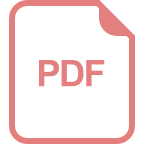
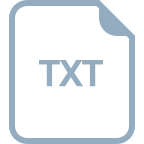
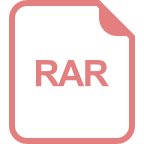
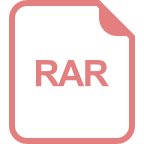
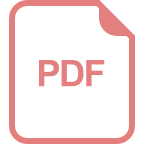
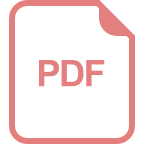
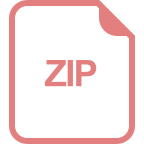
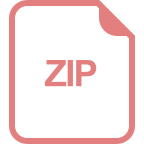