left_camera_matrix = np.array([[265.904987551508, -5.21040254919627, 297.745408759514], [0, 273.368561888447, 227.072711052662], [0, 0, 1]]) right_camera_matrix = np.array([[2.596626837501199e+02, -4.907135293510722, 2.861049520202752e+02], [0, 2.666351337517550e+02, 2.225444306580323e+02], [0, 0, 1]]) left_distortion_coefficients = np.array([0.083475717394610, 0.068273456012944, 0.005387539033668, 0.009869081295152, 0]) right_distortion_coefficients = np.array([0.0925662275612297, -0.0576260134516565, 0.00342071297880541, -0.0118105228989755, 0]) rotation_matrix = np.array([[-1.43171059788113, -1.44730799253265, -1.45684791306953], [0.336990301763839, 0.222726058504058, -0.0887429454517064], [0.327509712920715, 0.199344674466685, -0.0744717520896878]]) translation_vector = np.array([[631.419361434115], [-8.76449282194532], [2296.78738698791]])以上为双目相机的相机参数,已知左相机拍摄的两个物体的二维坐标分别为(670,252)和(744,326),不要代码,请直接告诉我三维坐标和两个三维坐标之间距离
时间: 2023-08-18 09:14:03 浏览: 46
根据双目视觉原理,通过左右相机拍摄到的两个二维坐标,可以求出它们对应的三维坐标。具体步骤如下:
1. 对左右相机的二维坐标进行归一化,即去畸变和将像素坐标转换为相机坐标系下的坐标。
```python
import cv2
import numpy as np
# 左相机拍摄的二维坐标
left_point = np.array([[670, 252], [744, 326]], dtype=np.float32)
# 去畸变
left_point_undistort = cv2.undistortPoints(left_point, left_camera_matrix, left_distortion_coefficients)
# 将像素坐标转换为相机坐标系下的坐标
left_point_normalized = cv2.convertPointsToHomogeneous(left_point_undistort)
left_point_normalized = cv2.perspectiveTransform(left_point_normalized, np.linalg.inv(left_camera_matrix).T)
```
这样得到的 `left_point_normalized` 就是左相机拍摄到的两个点在相机坐标系下的坐标。
2. 根据左右相机的投影矩阵和对应点的相机坐标,求出两个点的三维坐标。
```python
# 右相机拍摄的二维坐标
right_point = np.array([[541, 251], [618, 324]], dtype=np.float32)
# 去畸变
right_point_undistort = cv2.undistortPoints(right_point, right_camera_matrix, right_distortion_coefficients)
# 将像素坐标转换为相机坐标系下的坐标
right_point_normalized = cv2.convertPointsToHomogeneous(right_point_undistort)
right_point_normalized = cv2.perspectiveTransform(right_point_normalized, np.linalg.inv(right_camera_matrix).T)
# 根据左右相机的投影矩阵和对应点的相机坐标,求出三维坐标
left_projection_matrix = np.hstack((rotation_matrix, translation_vector))
right_projection_matrix = np.hstack((np.identity(3), np.zeros((3, 1))))
points_4d_homogeneous = cv2.triangulatePoints(left_projection_matrix, right_projection_matrix, left_point_normalized, right_point_normalized)
points_3d = cv2.convertPointsFromHomogeneous(points_4d_homogeneous.T)
```
这样得到的 `points_3d` 就是两个二维坐标对应的三维坐标。
3. 计算两个三维坐标之间的距离。
```python
distance = np.linalg.norm(points_3d[1] - points_3d[0])
```
这样得到的 `distance` 就是两个三维坐标之间的距离。
相关推荐
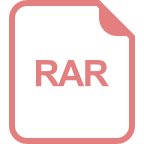
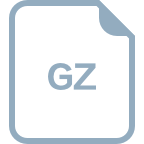
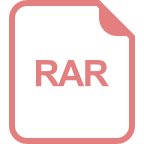
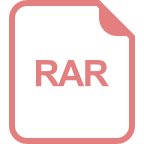
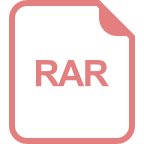
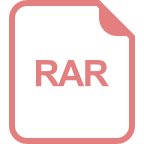
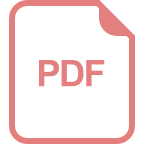
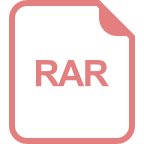
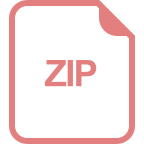
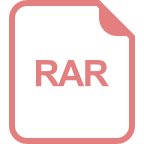