如何使用JPA的Specification实现三表连接模糊查询,并写出示例代码
时间: 2023-08-31 18:23:58 浏览: 63
### 回答1:
可以使用JPA的Specification实现三表连接模糊查询,示例代码如下:
```
public Specification<User> fuzzyQuery(String keyword) {
return (root, query, cb) -> {
Join<User, Order> orderJoin = root.join("orders", JoinType.LEFT);
Join<Order, Product> productJoin = orderJoin.join("products", JoinType.LEFT);
Predicate predicate = cb.like(root.get("name"), "%" + keyword + "%");
predicate = cb.or(predicate, cb.like(orderJoin.get("orderNo"), "%" + keyword + "%"));
predicate = cb.or(predicate, cb.like(productJoin.get("name"), "%" + keyword + "%"));
return predicate;
};
}
```
这段代码实现了对User、Order、Product三张表的连接查询,并且可以根据关键字进行模糊查询。其中,root表示User表的根节点,orderJoin表示User表与Order表的连接,productJoin表示Order表与Product表的连接。使用cb.like方法实现模糊查询,最后返回Predicate对象。
### 回答2:
在使用JPA的Specification实现三表连接模糊查询时,可以按照以下步骤进行操作:
1. 创建实体类:首先创建需要查询的三个实体类,并在实体类中定义三个表之间的关联关系。
2. 继承Specification接口:创建一个自定义的Specification接口,该接口需要继承JpaSpecificationExecutor接口,用于实现Specification的各种查询条件。
3. 实现Specification的方法:在自定义的Specification接口中,实现toPredicate方法,用来设置各种查询条件和连接条件。该方法需要返回一个Predicate对象,该对象将作为查询条件传递给JpaSpecificationExecutor接口。
4. 使用Specification进行查询:在使用JpaRepository时,可以使用Specification对象进行查询。示例代码如下:
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long>, JpaSpecificationExecutor<User> {
}
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> searchUsers(String keyword) {
Specification<User> spec = new Specification<User>() {
@Override
public Predicate toPredicate(Root<User> root, CriteriaQuery<?> query, CriteriaBuilder cb) {
Join<User, Order> orderJoin = root.join("orders", JoinType.INNER);
Join<Order, Product> productJoin = orderJoin.join("product", JoinType.INNER");
Predicate p1 = cb.like(root.get("name"), "%" + keyword + "%");
Predicate p2 = cb.like(orderJoin.get("orderId"), "%" + keyword + "%");
Predicate p3 = cb.like(productJoin.get("productName"), "%" + keyword + "%");
return cb.or(p1, p2, p3);
}
};
return userRepository.findAll(spec);
}
}
```
在上述示例中,首先使用Specification接口实现了toPredicate方法,在该方法中定义了三个表之间的连接关系和模糊查询的条件。然后在UserService中注入了UserRepository,并使用findAll方法传入Specification对象进行查询操作。
通过以上步骤,我们可以使用JPA的Specification实现三表连接模糊查询,根据需求定义查询条件和连接关系,灵活地查询数据。
### 回答3:
使用JPA的Specification实现三表连接模糊查询可以通过以下步骤实现:
1.定义三个实体类,分别表示三个表,假设为表A、表B和表C。
2.在每个实体类中定义对应的属性和关联关系,例如表A中有一个外键关联到表B,表B中有一个外键关联到表C。
3.创建一个自定义的Specification类,继承JpaSpecificationExecutor接口,并实现Specification接口。
4.在自定义的Specification类中重写toPredicate方法,通过CriteriaBuilder构建查询条件,使用root进行连接查询和模糊查询。
5.在业务层中调用Specification定义的方法,传入条件参数并调用repository的findAll方法即可实现查询。
示例代码如下:
```java
@Entity
@Table(name = "table_a")
public class TableA {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "table_b_id")
private TableB tableB;
// getters and setters
}
@Entity
@Table(name = "table_b")
public class TableB {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "type")
private String type;
@OneToMany(mappedBy = "tableB", cascade = CascadeType.ALL)
private List<TableA> tableAList;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "table_c_id")
private TableC tableC;
// getters and setters
}
@Entity
@Table(name = "table_c")
public class TableC {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "title")
private String title;
@OneToMany(mappedBy = "tableC", cascade = CascadeType.ALL)
private List<TableB> tableBList;
// getters and setters
}
public class MySpecification implements Specification<TableA> {
private String keyword;
public MySpecification(String keyword) {
this.keyword = keyword;
}
@Override
public Predicate toPredicate(Root<TableA> root, CriteriaQuery<?> criteriaQuery, CriteriaBuilder criteriaBuilder) {
Join<TableA, TableB> joinAB = root.join("tableB");
Join<TableB, TableC> joinBC = joinAB.join("tableC");
return criteriaBuilder.or(
criteriaBuilder.like(root.get("name"), "%" + keyword + "%"),
criteriaBuilder.like(joinAB.get("type"), "%" + keyword + "%"),
criteriaBuilder.like(joinBC.get("title"), "%" + keyword + "%")
);
}
}
public class MyService {
@Autowired
private TableARepository tableARepository;
public List<TableA> search(String keyword) {
MySpecification specification = new MySpecification(keyword);
return tableARepository.findAll(specification);
}
}
```
以上示例代码中的TableARepository为自定义的JpaRepository,在其继承的JpaRepository类中已经包含了JpaSpecificationExecutor接口,因此可以直接调用findAll方法并传入自定义的Specification实现类进行查询。在MyService中调用MySpecification定义的方法,并传入查询关键字,即可进行三表连接模糊查询。
相关推荐
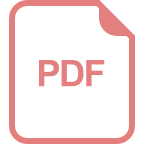
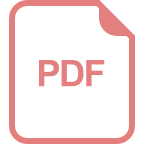














