按照下列要求编写程序 (1)从文件中读取数据流,数据均为整数; (2)设置时间语义为EventTime, 定义滚动窗口,窗口宽度10s; (3)对窗口内的数据求和; (4)结果保存到文件中。
时间: 2023-11-28 15:47:50 浏览: 99
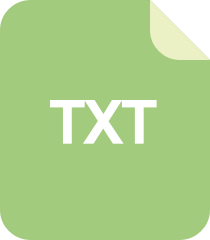
用C++编写从文件中读取数据的程序

以下是使用 Apache Flink 编写的程序。假设输入文件中每行只包含一个整数,且文件路径为 "input.txt",输出结果保存到文件 "output.txt"。
```java
import org.apache.flink.api.common.functions.ReduceFunction;
import org.apache.flink.api.java.functions.KeySelector;
import org.apache.flink.api.java.tuple.Tuple;
import org.apache.flink.api.java.tuple.Tuple1;
import org.apache.flink.api.java.utils.ParameterTool;
import org.apache.flink.streaming.api.TimeCharacteristic;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.functions.windowing.WindowFunction;
import org.apache.flink.streaming.api.windowing.assigners.TumblingEventTimeWindows;
import org.apache.flink.streaming.api.windowing.time.Time;
import org.apache.flink.streaming.api.windowing.windows.TimeWindow;
import org.apache.flink.streaming.connectors.fs.FileSystem;
import org.apache.flink.util.Collector;
import java.util.Iterator;
public class EventTimeRollingSum {
public static void main(String[] args) throws Exception {
// 解析命令行参数
final ParameterTool params = ParameterTool.fromArgs(args);
// 获取执行环境
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
// 设置时间语义为 EventTime
env.setStreamTimeCharacteristic(TimeCharacteristic.EventTime);
// 读取输入数据
DataStream<Integer> input = env.readTextFile(params.get("input"))
.map(Integer::parseInt)
.assignTimestampsAndWatermarks(new CustomTimestampExtractor()); // 自定义 TimestampExtractor
// 定义窗口并计算每个窗口内的数据总和
DataStream<Tuple1<Integer>> output = input
.keyBy((KeySelector<Integer, Tuple>) value -> Tuple1.of(0)) // 将所有数据分到同一个组
.window(TumblingEventTimeWindows.of(Time.seconds(10))) // 定义滚动窗口,窗口宽度为 10s
.apply(new WindowFunction<Integer, Tuple1<Integer>, Tuple, TimeWindow>() { // 计算窗口内的数据总和
@Override
public void apply(Tuple key, TimeWindow window, Iterable<Integer> input, Collector<Tuple1<Integer>> out) throws Exception {
int sum = 0;
Iterator<Integer> iterator = input.iterator();
while (iterator.hasNext()) {
sum += iterator.next();
}
out.collect(Tuple1.of(sum));
}
});
// 将结果保存到文件
output.writeAsText(params.get("output"), FileSystem.WriteMode.OVERWRITE);
// 执行程序
env.execute("EventTimeRollingSum");
}
private static class CustomTimestampExtractor implements org.apache.flink.streaming.api.functions.timestamps.TimestampExtractor<Integer> {
@Override
public long extractTimestamp(Integer element, long recordTimestamp) {
return System.currentTimeMillis(); // 使用系统时间作为 EventTime
}
}
}
```
需要注意以下几点:
1. 程序中使用了自定义的 `CustomTimestampExtractor` 来指定 EventTime。在这个例子中,我们将 EventTime 设置为系统时间,实际生产中需要根据业务需求来设置。
2. 在 `keyBy` 操作中,将所有数据分到同一个组中,这样所有数据都会进入同一个窗口。
3. 在窗口计算中,使用了 `WindowFunction` 和 `ReduceFunction`。其中 `WindowFunction` 用于计算窗口内的数据总和,`ReduceFunction` 用于在窗口外进行数据合并。在这个例子中,我们只需要使用 `WindowFunction`。
阅读全文
相关推荐
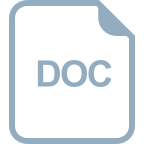
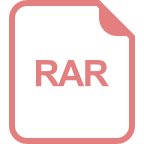
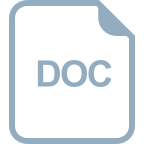
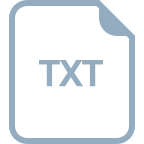
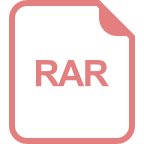
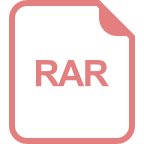
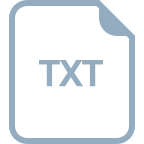
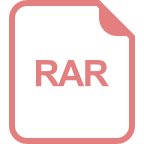
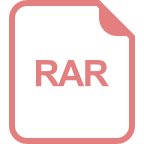
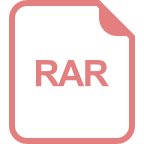
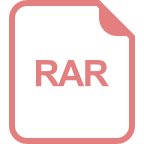
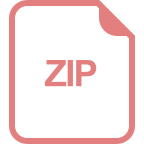
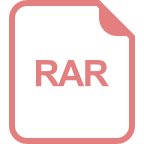