uniapp小程序如何获取某个相对定位元素距离底部的高度,请使用vue3写法
时间: 2023-12-02 10:06:07 浏览: 43
好的,以下是使用 Vue3 在 uniapp 小程序中获取某个相对定位元素距离底部的高度的写法:
1. 在模板中使用 `ref` 给元素添加引用。
```html
<template>
<div ref="myElement" style="position: relative; bottom: 0;">Hello World</div>
</template>
```
2. 在组件中使用 `onMounted` 生命周期钩子函数获取元素引用,并使用 `createApp` 函数创建应用实例,通过 `directive` 注册自定义指令 `relative`。
```javascript
<script>
import { onMounted, createApp } from 'vue'
export default {
setup() {
const myElement = ref(null)
onMounted(() => {
// 获取元素引用
const element = myElement.value
// 创建应用实例
const app = createApp({})
// 注册自定义指令
app.directive('relative', {
mounted(el, binding) {
const callback = binding.value
const options = { threshold: 0.5 }
const observer = new IntersectionObserver(([entry]) => {
if (entry.isIntersecting) {
observer.unobserve(element)
const bottom = entry.boundingClientRect.bottom
callback(bottom)
}
}, options)
observer.observe(element)
}
})
})
return {
myElement
}
}
}
</script>
```
在上述代码中,我们首先使用 `onMounted` 生命周期钩子函数获取元素引用 `myElement`。接着,使用 `createApp` 函数创建应用实例,并通过 `directive` 方法注册自定义指令 `relative`,该指令使用 `IntersectionObserver` 监听元素是否进入视口,并在元素进入视口时执行回调函数,并传递元素底部距离作为参数。
3. 在模板中使用自定义指令 `v-relative` 并传入回调函数。
```html
<template>
<div ref="myElement" style="position: relative; bottom: 0;" v-relative="onRelative">Hello World</div>
</template>
```
在上述代码中,我们使用自定义指令 `v-relative` 绑定元素,并传入回调函数 `onRelative`,该函数将在元素进入视口时被调用,并将元素底部距离作为参数传递。
需要注意的是,在 Vue3 中,我们需要使用 `createApp` 函数创建应用实例,并将自定义指令注册到应用实例中。同时,由于 Vue3 中使用了 Composition API,因此需要使用 `setup` 函数来管理组件的状态和行为。
相关推荐
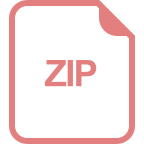














