建立一个对象数组,内放5个学生的数据(学号、成绩),设立一个函数max,用指向对象的指针作函数参数,在max函数中找出5个学生中成绩最高者,并输出其学号。
时间: 2023-06-08 18:04:32 浏览: 78
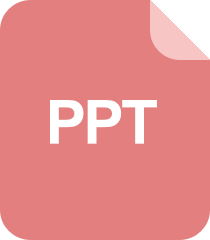
指向结构体数组的指针-C语言入门教程
好的,这是一个关于 C++ 编程的问题。以下是代码实现:
```
#include <iostream>
using namespace std;
class Student {
public:
int number;
int score;
};
void max(Student *stu[]) {
int maxScore = 0;
int maxIndex = -1;
for (int i = 0; i < 5; i++) {
if (stu[i]->score > maxScore) {
maxScore = stu[i]->score;
maxIndex = i;
}
}
cout << "成绩最高的学生学号是:" << stu[maxIndex]->number << endl;
}
int main() {
Student *stu[5];
for (int i = 0; i < 5; i++) {
stu[i] = new Student();
cout << "请输入第 " << i+1 << " 个学生的学号和成绩:";
cin >> stu[i]->number >> stu[i]->score;
}
max(stu);
for (int i = 0; i < 5; i++) {
delete stu[i];
}
return 0;
}
```
解释一下上面的代码,我们首先定义了一个 `Student` 类,包括学号和成绩两个属性。然后在 `max` 函数中,我们使用指针数组 `stu` 来存储 5 个学生的指针,遍历这个数组,找到成绩最高的学生,并输出其学号。在 `main` 函数中,我们先用 `new` 命令分别为 5 个学生动态分配内存,并让指针数组 `stu` 指向它们,然后用 `cin` 命令从输入流中读取学号和成绩。之后调用 `max` 函数,找到成绩最高的学生并输出学号。最后,我们再用 `delete` 命令释放掉动态分配的内存。
阅读全文
相关推荐
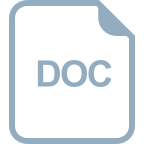
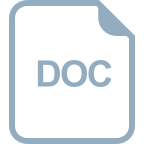










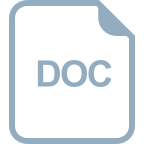
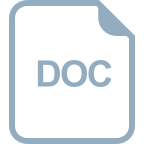
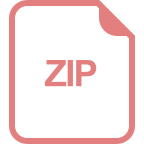