Qtablewidget表格中添加单选框 c++代码实现
时间: 2023-08-27 12:03:48 浏览: 156
可以使用自定义委托来实现在QTableWidget中添加单选框。下面是一个简单的示例代码:
1. 创建一个自定义委托类:
```cpp
class RadioButtonDelegate : public QStyledItemDelegate {
public:
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override {
Q_UNUSED(option);
if (index.column() == 0) {
QRadioButton *radioButton = new QRadioButton(parent);
return radioButton;
} else {
return QStyledItemDelegate::createEditor(parent, option, index);
}
}
void setEditorData(QWidget *editor, const QModelIndex &index) const override {
if (QRadioButton *radioButton = qobject_cast<QRadioButton *>(editor)) {
bool value = index.model()->data(index, Qt::EditRole).toBool();
radioButton->setChecked(value);
} else {
QStyledItemDelegate::setEditorData(editor, index);
}
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override {
if (QRadioButton *radioButton = qobject_cast<QRadioButton *>(editor)) {
model->setData(index, radioButton->isChecked(), Qt::EditRole);
} else {
QStyledItemDelegate::setModelData(editor, model, index);
}
}
void updateEditorGeometry(QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const override {
Q_UNUSED(index);
editor->setGeometry(option.rect);
}
};
```
2. 在QTableWidget中设置委托:
```cpp
QTableWidget *tableWidget = new QTableWidget(this);
tableWidget->setRowCount(3);
tableWidget->setColumnCount(2);
// 设置第一列为单选框
tableWidget->setItemDelegateForColumn(0, new RadioButtonDelegate());
// 添加表格项
for (int row=0; row<3; row++) {
QTableWidgetItem *item1 = new QTableWidgetItem();
item1->setFlags(item1->flags() & ~Qt::ItemIsEditable); // 第一列不可编辑
tableWidget->setItem(row, 0, item1);
QTableWidgetItem *item2 = new QTableWidgetItem(QString("Item %1").arg(row));
tableWidget->setItem(row, 1, item2);
}
```
这样就可以在QTableWidget的第一列中添加单选框了。
阅读全文
相关推荐
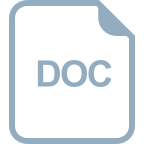
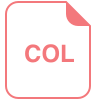

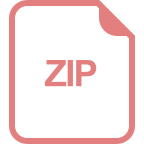
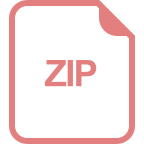
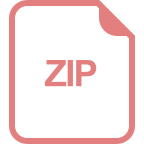