vue 高德地图叠加手绘图
时间: 2023-07-05 13:30:52 浏览: 128
要在 Vue 中叠加手绘图到高德地图中,可以使用高德地图 JavaScript API 中的 Overlay 类来实现。具体步骤如下:
1. 在 Vue 项目中引入高德地图 JavaScript API 的 SDK 文件,可以通过 npm 安装或者使用 script 标签引入。
2. 在 Vue 组件的 mounted 钩子函数中,创建一个地图实例并初始化。
3. 创建一个自定义 Overlay 类,继承自 AMap.Overlay 类。在该类中实现 draw 方法来绘制手绘图。
4. 在地图的 add 方法中添加自定义 Overlay 实例,即可将手绘图叠加到地图中。
下面是一个简单的示例代码:
```
<template>
<div id="map" style="height: 500px;"></div>
</template>
<script>
import AMapLoader from '@amap/amap-jsapi-loader'
export default {
name: 'AmapDemo',
data() {
return {
map: null,
}
},
mounted() {
AMapLoader.load({
key: 'your amap api key',
version: '2.0',
plugins: ['AMap.Scale', 'AMap.OverView', 'AMap.ToolBar', 'AMap.MapType'],
}).then((AMap) => {
this.map = new AMap.Map('map', {
zoom: 12,
center: [116.397428, 39.90923],
})
const MyOverlay = function () {
this.draw = function () {
const context = this.getContext('2d')
context.beginPath()
context.moveTo(100, 100)
context.lineTo(200, 200)
context.stroke()
}
}
MyOverlay.prototype = new AMap.Overlay()
MyOverlay.prototype.constructor = MyOverlay
MyOverlay.prototype.onAdd = function () {
const canvas = document.createElement('canvas')
canvas.width = this.getMap().getSize().getWidth()
canvas.height = this.getMap().getSize().getHeight()
this.setCanvas(canvas)
const panes = this.getPanes()
panes.overlayLayer.appendChild(canvas)
}
MyOverlay.prototype.onRemove = function () {
this.getCanvas().parentNode.removeChild(this.getCanvas())
}
const myOverlay = new MyOverlay()
this.map.add(myOverlay)
})
},
}
</script>
```
在上面的示例中,我们首先引入了 AMapLoader 库来加载高德地图的 SDK 文件。在 mounted 钩子函数中,我们创建了一个地图实例,并使用自定义 Overlay 类 MyOverlay 来绘制手绘图。在 MyOverlay 类的 draw 方法中,我们使用 Canvas API 来绘制一条从 (100, 100) 到 (200, 200) 的直线。最后,在地图的 add 方法中添加 MyOverlay 实例,即可将手绘图叠加到地图中。
相关推荐
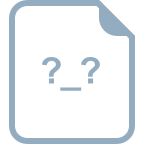














