用c语言编写对一个整型数组a[n]编程实现一组排序算法,要求利用随机函数生成1000个随机数,存放到数组中;分析算法时间复杂度;测试程序执行时间
时间: 2024-09-13 21:16:20 浏览: 56
在C语言中,可以使用各种排序算法对整型数组进行排序。这里我将提供一个使用快速排序算法的示例,并说明如何利用随机函数生成随机数填充数组,分析算法的时间复杂度以及测试程序执行时间的方法。
首先,快速排序算法的基本思想是:选择一个基准元素,重新排列数组,使得所有比基准小的元素放在基准前面,所有比基准大的元素放在基准后面。在这个分区退出之后,该基准就处于数组的中间位置。递归地在基准左边和右边的子数组上重复这个过程。
以下是快速排序算法的一个实现示例,包括随机数生成和时间复杂度分析:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 1000
void quickSort(int arr[], int low, int high);
int partition(int arr[], int low, int high);
void swap(int* a, int* b);
void printArray(int arr[], int size);
int main() {
int a[SIZE];
int i;
// 初始化随机数生成器
srand((unsigned)time(NULL));
// 生成随机数并填充数组
for (i = 0; i < SIZE; i++) {
a[i] = rand() % 10000; // 假设生成的随机数范围是0到9999
}
// 打印未排序的数组
printf("Unsorted array:\n");
printArray(a, SIZE);
// 对数组进行快速排序
quickSort(a, 0, SIZE - 1);
// 打印排序后的数组
printf("Sorted array:\n");
printArray(a, SIZE);
return 0;
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
```
时间复杂度分析:
快速排序算法的平均时间复杂度是 O(n log n),在最好情况下也是 O(n log n),但如果数组已经是正序或逆序,则最坏情况下的时间复杂度会退化到 O(n^2)。快速排序的性能依赖于基准的选择,这里选择的是数组最后一个元素作为基准。
测试程序执行时间:
可以使用C语言中的`clock()`函数来测试程序的执行时间。`clock()`函数返回程序执行期间占用处理器的时钟周期数,可以用来粗略测量程序的执行时间。
```c
#include <stdio.h>
#include <time.h>
// ...之前的代码保持不变...
int main() {
// ...之前的代码保持不变...
// 记录开始时间
clock_t start = clock();
// 对数组进行快速排序
quickSort(a, 0, SIZE - 1);
// 记录结束时间
clock_t end = clock();
// 打印排序后的数组
printf("Sorted array:\n");
printArray(a, SIZE);
// 打印程序执行时间
printf("Execution time: %f seconds\n", (double)(end - start) / CLOCKS_PER_SEC);
return 0;
}
```
阅读全文
相关推荐
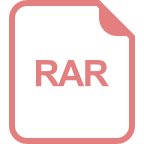
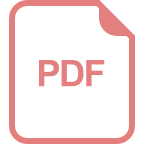
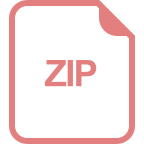

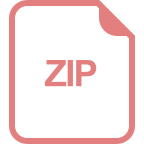
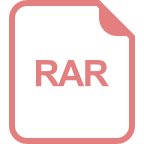
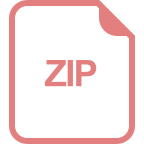
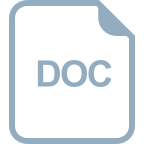
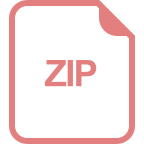
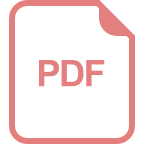
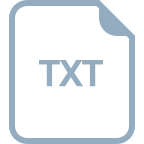
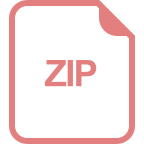
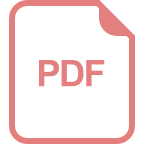
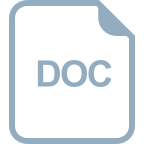
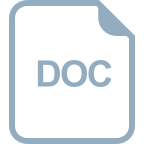
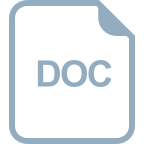
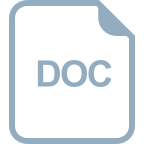
