(Java代码)编写具有图形用户界面(GUI)的日历组件显示日期和时间并进行适当 的功能扩充。 ·主要功能: 1、显示当月日历,当前日期、当前时间; 2、可查询任意月以及任意年的日历: 3、正常运行和退出程序。 4、每日具有记账功能,需要至少包含1)记账id:2)记账类型:3)支出费用;
时间: 2024-02-21 17:58:59 浏览: 89
以下是一个简单的Java代码,实现了具有图形用户界面的日历组件,并包含了上述主要功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class CalendarGUI extends JFrame implements ActionListener {
private JLabel timeLabel, dateLabel, monthLabel, yearLabel;
private JButton previousButton, nextButton;
private JComboBox<String> monthComboBox, yearComboBox;
private JTextArea accountTextArea;
private Calendar calendar;
public CalendarGUI() {
super("日历组件");
// 初始化日历
calendar = Calendar.getInstance();
// 初始化界面
initUI();
// 显示当前日期和时间
updateTime();
}
private void initUI() {
// 时间标签
timeLabel = new JLabel();
timeLabel.setFont(new Font("Arial", Font.PLAIN, 20));
add(timeLabel, BorderLayout.NORTH);
// 日期标签
dateLabel = new JLabel();
dateLabel.setFont(new Font("Arial", Font.PLAIN, 40));
dateLabel.setHorizontalAlignment(SwingConstants.CENTER);
add(dateLabel, BorderLayout.CENTER);
// 上一个月按钮
previousButton = new JButton("<");
previousButton.addActionListener(this);
add(previousButton, BorderLayout.WEST);
// 下一个月按钮
nextButton = new JButton(">");
nextButton.addActionListener(this);
add(nextButton, BorderLayout.EAST);
// 月份选择框
monthLabel = new JLabel("月份:");
add(monthLabel, BorderLayout.SOUTH);
String[] months = {"1", "2", "3", "4", "5", "6", "7", "8", "9", "10", "11", "12"};
monthComboBox = new JComboBox<>(months);
monthComboBox.setSelectedIndex(calendar.get(Calendar.MONTH));
monthComboBox.addActionListener(this);
add(monthComboBox, BorderLayout.SOUTH);
// 年份选择框
yearLabel = new JLabel("年份:");
add(yearLabel, BorderLayout.SOUTH);
String[] years = {"2020", "2021", "2022", "2023", "2024", "2025", "2026", "2027", "2028", "2029", "2030"};
yearComboBox = new JComboBox<>(years);
yearComboBox.setSelectedIndex(1);
yearComboBox.addActionListener(this);
add(yearComboBox, BorderLayout.SOUTH);
// 记账文本框
accountTextArea = new JTextArea(5, 20);
accountTextArea.setEditable(true);
add(new JScrollPane(accountTextArea), BorderLayout.SOUTH);
// 设置窗口大小和位置
setSize(600, 500);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
private void updateTime() {
// 更新时间标签
SimpleDateFormat timeFormat = new SimpleDateFormat("HH:mm:ss");
Date now = new Date();
timeLabel.setText(timeFormat.format(now));
// 更新日期标签
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy年MM月dd日 EEEE");
Date date = calendar.getTime();
dateLabel.setText(dateFormat.format(date));
}
private void updateCalendar() {
// 更新月份和年份
int month = monthComboBox.getSelectedIndex();
int year = Integer.parseInt((String) yearComboBox.getSelectedItem());
calendar.set(Calendar.MONTH, month);
calendar.set(Calendar.YEAR, year);
// 更新日期标签
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy年MM月dd日 EEEE");
Date date = calendar.getTime();
dateLabel.setText(dateFormat.format(date));
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == previousButton) {
// 上一个月
calendar.add(Calendar.MONTH, -1);
updateCalendar();
} else if (e.getSource() == nextButton) {
// 下一个月
calendar.add(Calendar.MONTH, 1);
updateCalendar();
} else if (e.getSource() == monthComboBox || e.getSource() == yearComboBox) {
// 选择月份或年份
updateCalendar();
}
}
public static void main(String[] args) {
new CalendarGUI();
}
}
```
代码中使用了Swing库来创建用户界面,包含了时间标签、日期标签、上一个月按钮、下一个月按钮、月份选择框、年份选择框和记账文本框。其中,时间和日期标签会随着时间和日期的变化而动态更新。通过上一个月和下一个月按钮或者月份选择框和年份选择框,用户可以查看任意月份的日历。同时,记账文本框可以记录每天的支出费用。
阅读全文
相关推荐
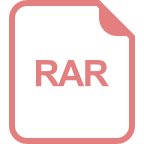
















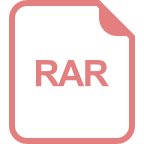