使用多线程处理文件分片的上传逻辑
时间: 2023-08-30 17:07:09 浏览: 194
当使用多线程处理文件分片的上传逻辑时,可以使用Python的`concurrent.futures`模块来实现线程池和并发执行任务的功能。以下是一个示例代码:
```python
import os
import tornado.ioloop
import tornado.web
from concurrent.futures import ThreadPoolExecutor
class UploadHandler(tornado.web.RequestHandler):
executor = ThreadPoolExecutor(max_workers=4)
@tornado.web.asynchronous
@tornado.gen.coroutine
def post(self):
file = self.request.files['file'][0]
filename = file['filename']
temp_path = os.path.join('temp', filename)
with open(temp_path, 'wb') as f:
f.write(file['body'])
# 处理文件分片的上传逻辑,使用线程池进行并发处理
future = self.executor.submit(self.process_file, temp_path)
tornado.ioloop.IOLoop.current().add_future(future, self.on_complete)
def process_file(self, temp_path):
# 在这里实现文件分片上传的逻辑
# 可以使用多线程进行并发处理
# 示例:将分片文件合并为完整的文件
output_file = 'output.txt'
with open(output_file, 'ab') as output:
with open(temp_path, 'rb') as f:
output.write(f.read())
# 删除临时文件
os.remove(temp_path)
def on_complete(self, future):
# 文件上传完成后的回调函数
self.finish("Upload complete")
def make_app():
return tornado.web.Application([
(r"/upload", UploadHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8888)
tornado.ioloop.IOLoop.current().start()
```
在上述代码中,我们使用`ThreadPoolExecutor`创建了一个具有4个工作线程的线程池。在`post`方法中,我们将文件分片保存到临时文件夹后,使用线程池的`submit`方法提交了一个任务,该任务会调用`process_file`方法来处理文件分片的上传逻辑。`process_file`方法中,我们可以使用多线程进行并发处理,例如将分片文件合并为完整的文件。最后,在任务完成后的回调函数`on_complete`中,我们发送响应给客户端,表示文件上传完成。
请注意,在实际应用中,可能需要添加更多的错误处理、并发控制以及对临时文件的加锁操作,以确保线程安全性和文件上传的可靠性。
阅读全文
相关推荐
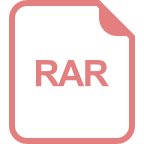
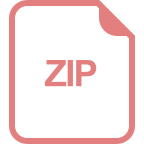
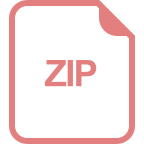
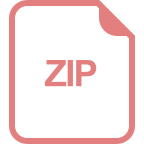
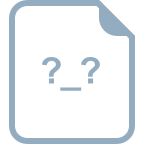
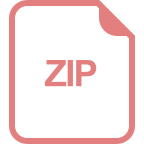
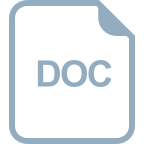
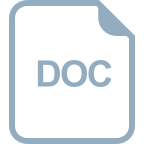
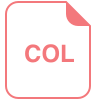
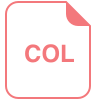

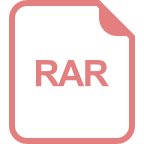
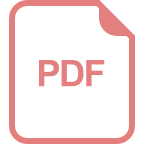
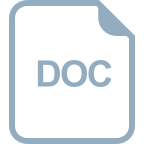
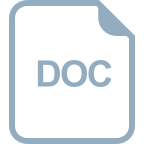
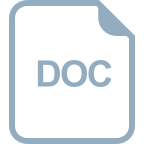
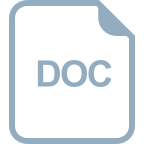