python 多线程并发登录
时间: 2023-06-14 18:07:58 浏览: 78
可以使用 Python 的 threading 和 requests 库实现多线程并发登录。具体步骤如下:
1. 导入 threading 和 requests 库:
```
import threading
import requests
```
2. 创建一个登录函数 login,用来实现登录逻辑:
```
def login(username, password):
# 构造登录请求
url = "http://example.com/login"
data = {
"username": username,
"password": password
}
response = requests.post(url, data=data)
# 处理登录结果
if response.status_code == 200:
print(f"{username} 登录成功")
else:
print(f"{username} 登录失败")
```
3. 创建一个线程类 LoginThread,用来实现多线程登录:
```
class LoginThread(threading.Thread):
def __init__(self, username, password):
threading.Thread.__init__(self)
self.username = username
self.password = password
def run(self):
login(self.username, self.password)
```
4. 创建多个 LoginThread 实例并启动线程:
```
thread1 = LoginThread("user1", "password1")
thread2 = LoginThread("user2", "password2")
thread3 = LoginThread("user3", "password3")
thread1.start()
thread2.start()
thread3.start()
thread1.join()
thread2.join()
thread3.join()
```
以上就是使用 Python 实现多线程并发登录的简单示例,可以根据实际需要进行调整。需要注意的是,如果登录请求涉及到验证码等复杂逻辑,需要对登录函数进行相应的修改。
相关推荐
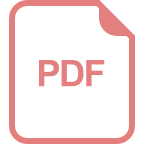
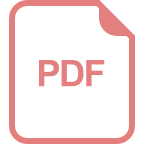














