在JupyterLab上对一张灰度图像进行基于DWT的图像压缩的代码,要求能控制压缩比
时间: 2024-02-20 07:57:56 浏览: 78
下面是基于DWT的图像压缩代码,可以在JupyterLab上运行。其中使用了pywt库进行DWT变换。
```python
import numpy as np
import matplotlib.pyplot as plt
import pywt
from skimage import io, color
# 读取灰度图像
img = color.rgb2gray(io.imread('lena.png'))
# 对图像进行DWT变换
coeffs = pywt.dwt2(img, 'haar')
# 设置压缩比率
compress_ratio = 0.5
# 计算阈值
threshold = np.sort(np.abs(coeffs[1].ravel()))[::-1][int(compress_ratio * len(coeffs[1].ravel()))]
# 对小于阈值的系数进行置零
coeffs = list(coeffs)
coeffs[0] = np.round(coeffs[0])
coeffs[1] = np.where(np.abs(coeffs[1]) < threshold, 0, coeffs[1])
coeffs[2] = np.where(np.abs(coeffs[2]) < threshold, 0, coeffs[2])
# 对图像进行IDWT反变换
img_dwt = pywt.idwt2(coeffs, 'haar')
# 显示原始图像和压缩后的图像
fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(8, 4))
ax = axes.ravel()
ax[0].imshow(img, cmap=plt.cm.gray)
ax[0].set_title("Original image")
ax[1].imshow(img_dwt, cmap=plt.cm.gray)
ax[1].set_title("Compressed image (DWT)")
ax[1].set_xlabel("Compression ratio: {:.2f}".format(compress_ratio))
plt.tight_layout()
plt.show()
```
代码中首先读取灰度图像,并对图像进行DWT变换。然后通过设置压缩比率,计算出阈值,并将小于阈值的系数置零。最后对图像进行IDWT反变换,得到压缩后的图像,并将原始图像和压缩后的图像进行显示比较。
需要注意的是,代码中使用了skimage库中的color.rgb2gray函数将RGB图像转换为灰度图像,如果输入的是灰度图像则不需要进行转换。同时,需要将图像的RGB值转换为灰度值,即将RGB值的平均值作为灰度值。
阅读全文
相关推荐


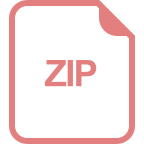
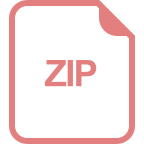
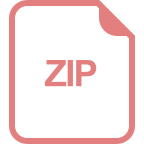
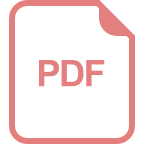
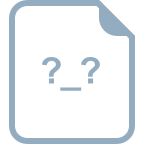
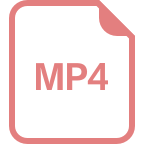
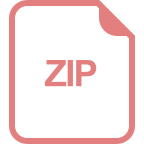
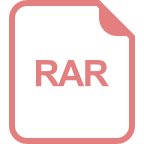
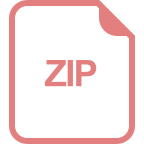
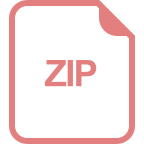
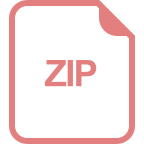
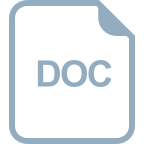
