面向对象的思想实现排序算法 代码
时间: 2023-08-12 17:58:09 浏览: 133
当使用面向对象的思想实现排序算法时,通常将排序算法封装为一个类,其中包含排序函数以及其他必要的辅助函数。下面是一个使用面向对象思想实现的冒泡排序算法的示例代码:
```cpp
#include <iostream>
#include <vector>
class BubbleSort {
public:
static void sort(std::vector<int>& arr) {
int n = arr.size();
for (int i = 0; i < n-1; ++i) {
for (int j = 0; j < n-i-1; ++j) {
if (arr[j] > arr[j+1]) {
std::swap(arr[j], arr[j+1]);
}
}
}
}
};
int main() {
std::vector<int> nums = {4, 2, 6, 8, 1, 3, 5, 7};
BubbleSort::sort(nums);
std::cout << "Sorted array: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
上述代码中,我们创建了一个名为`BubbleSort`的类,其中包含一个名为`sort`的静态成员函数。该函数接受一个整数向量作为输入,并使用冒泡排序算法对其进行排序。在`main`函数中,我们创建一个整数向量`nums`并调用`BubbleSort::sort`函数对其进行排序,然后输出排序后的结果。
需要注意的是,这只是一个简单的示例,你可以根据需要实现其他排序算法,或在排序类中添加其他功能函数。
阅读全文
相关推荐
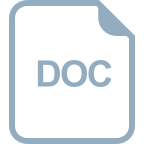
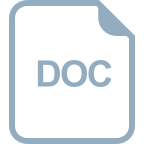
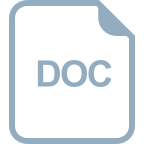
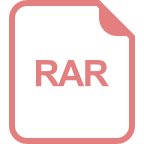
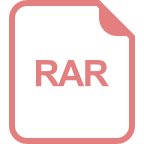
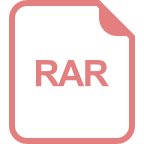
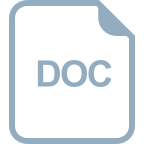
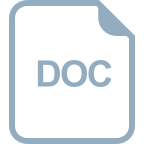
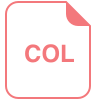
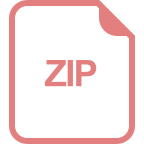
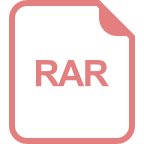
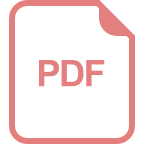
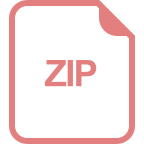
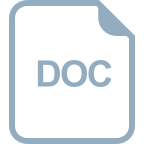
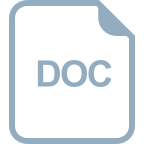
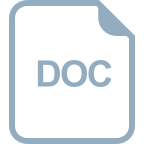
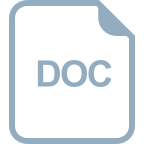
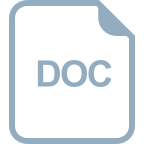
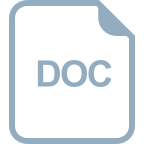