error CS0266: 无法将类型“System.Linq.IOrderedEnumerable<System.Collections.Generic.KeyValuePair<int, string>>”隐式转换为“System.Collections.Generic.Dictionary<int, string>”。存在一个显式转换(是否缺少强制转换?) 1>E:\TSZNet\TszTool\trunk\AttendanceManager\AttendanceManager\SRedmineInstance.cs(213,24,213,25): warning CS0168: 声明了变量“e”,但从未使用过 1>E:\TSZNet\TszTool\trunk\AttendanceManager\AttendanceManager\SRedmineInstance.cs(232,24,232,25): warning CS0168: 声明了变量“e”,但从未使用过
时间: 2023-07-21 19:11:07 浏览: 43
这个错误提示是因为 OrderBy 方法返回的是一个 IOrderedEnumerable<KeyValuePair<int, string>> 类型的序列,而不是一个 Dictionary<int, string> 类型的字典。如果你需要将排序后的结果转换为字典类型,可以使用 ToDictionary 方法将序列转换为字典类型,示例如下:
```csharp
Dictionary<int, string> dict = new Dictionary<int, string>();
dict.Add(3, "three");
dict.Add(1, "one");
dict.Add(2, "two");
var sortedDict = dict.OrderBy(x => x.Key)
.ToDictionary(x => x.Key, x => x.Value);
foreach (var item in sortedDict)
{
Console.WriteLine(item.Key + ": " + item.Value);
}
```
上述代码将按照字典键的升序对 Dictionary<int, string> 进行排序,并使用 ToDictionary 方法将排序后的序列转换为字典类型,输出每个键值对。
相关问题
无法将类型“Newtonsoft.Json.Linq.JToken”隐式转换为“System.Collections.Generic.List<Newtonsoft.Json.Linq.JProperty>
这个错误提示是因为您试图将类型为 `JToken` 的对象转换为 `List<JProperty>` 类型,但是这两个类型之间是无法隐式转换的。
解决方案:
您需要将 `JToken` 对象转换为 `JObject` 类型,然后再通过 `Properties()` 方法获取到 `List<JProperty>` 对象。示例如下:
```csharp
JToken token = JToken.Parse(jsonString); // 从字符串解析出 JToken 对象
JObject jObject = token as JObject; // 将 JToken 对象转换为 JObject 类型
List<JProperty> properties = jObject.Properties().ToList(); // 获取 JObject 对象的属性列表
```
注意:在使用 `as` 进行类型转换时,需要注意对象是否为 null,否则会抛出空引用异常。
C#报找不到方法:“System.Collections.Generic.IEnumerator`1<Newtonsoft.Json.Linq.JToken> Newtonsoft.Json.Linq.JArray.GetEnumerator()”
在C#中,报错"找不到方法"通常是由于以下几种情况引起的:
1. 方法不存在:检查代码中是否正确引用了相关的命名空间和程序集。确保你已经正确地添加了对Newtonsoft.Json的引用,并且版本匹配。
2. 方法签名不匹配:检查方法的参数类型和返回类型是否与调用代码中的期望一致。在这种情况下,报错可能是由于方法签名不匹配导致的。
3. 版本冲突:如果你的项目中同时引用了多个版本的Newtonsoft.Json,可能会导致方法找不到的问题。请确保你的项目只引用了一个版本,并且与你使用的代码兼容。
解决这个问题的方法包括:
1. 确认你的项目引用了正确的Newtonsoft.Json版本,并且与你使用的代码兼容。
2. 检查方法调用的代码,确保参数类型和返回类型与方法定义一致。
3. 如果可能,尝试更新Newtonsoft.Json到最新版本,以确保解决了任何已知的问题。
4. 如果以上方法都没有解决问题,可以尝试重新构建项目,清理并重新生成解决方案。
相关推荐
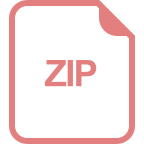












