用Python爬虫爬取古诗文网中推荐栏目的数据
时间: 2024-11-04 22:15:32 浏览: 61
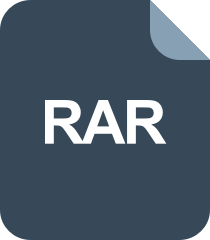
python爬虫爬古诗文网的数据
在Python中,使用爬虫技术抓取古诗文网(www.gushiwen.org)的推荐栏目数据通常需要以下几个步骤:
1. **选择库**:首先,你需要安装一些必要的Python库,如`requests`用于发送HTTP请求,`BeautifulSoup`或`lxml`处理HTML文档,以及`pandas`用于数据分析。
```python
pip install requests beautifulsoup4 pandas
```
2. **发送请求**:使用`requests.get()`函数向网站发送GET请求,并获取网页内容。
```python
import requests
url = "https://www.gushiwen.org/zige/"
response = requests.get(url)
html_content = response.text
```
3. **解析HTML**:通过BeautifulSoup解析HTML内容,找到包含推荐信息的部分,这通常涉及到查找特定的标签或CSS类名。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
recommendation_blocks = soup.find_all('div', class_='list-item') # 或者其他的标签名
```
4. **提取数据**:遍历找到的区块,提取你需要的数据,比如标题、作者、链接等。你可以使用`.find()`或`.get_text()`等方法。
```python
data = []
for block in recommendation_blocks:
title = block.find('a', class_='title').text
author = block.find('span', class_='author').text
link = block.find('a')['href']
data.append({
'title': title,
'author': author,
'link': 'https://www.gushiwen.org' + link, # 合并URL以形成完整地址
})
```
5. **保存数据**:将爬取到的数据存储到CSV文件或数据库中,以便后续分析。
```python
import pandas as pd
df = pd.DataFrame(data)
df.to_csv('gushiwen_recommendations.csv', index=False)
```
6. **遵守规则**:在进行网络爬虫时,请务必遵守网站的robots.txt协议,尊重版权,避免对服务器造成过大压力。
注意:由于古诗文网或其他网站可能会有反爬虫机制或改变页面结构,以上代码可能需要根据实际网站情况进行调整。同时,爬虫操作应合法合规,保护个人隐私和他人权益。
阅读全文
相关推荐
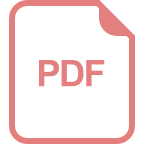
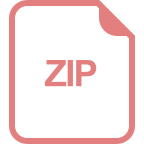
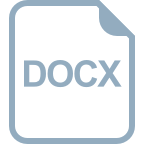
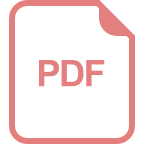
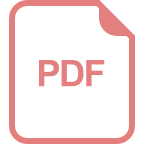
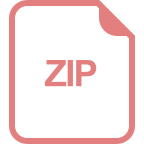
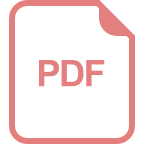
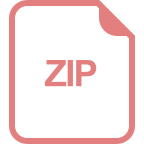
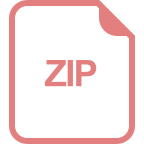
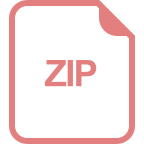
