请实现一个如图所示的Windows窗体应用,使用到的控件是:ComboBox(下拉列表),button(按钮), Label(标签)。实现的功能为:点击【抽奖】,从所选择的抽奖范围中随机抽取一个人,下方的label展示抽奖结果。
时间: 2024-09-09 19:14:46 浏览: 107
要实现这样一个Windows窗体应用,你需要使用一个Windows窗体设计器,比如在Visual Studio中进行操作。以下是基本步骤和代码示例:
1. 打开Visual Studio,创建一个新的Windows窗体应用程序项目。
2. 在工具箱中找到ComboBox、Button和Label控件,将它们拖放到窗体上。
3. 设置ComboBox控件的属性,例如`Name`属性,以及添加一些初始选项,这些选项代表抽奖的范围。
4. 设置Button控件的`Name`属性,并将其`Text`属性设置为“抽奖”。
5. 设置Label控件的`Name`属性,用来显示抽奖结果。
接下来,你需要为Button控件的Click事件添加一个事件处理方法。在这个方法中,你将使用ComboBox控件的当前选中项作为抽奖范围,然后生成一个随机数来从这个范围中选择一个随机项,并将其显示在Label控件上。
以下是C#代码示例:
```csharp
using System;
using System.Windows.Forms;
namespace WindowsFormsApp
{
public partial class MainForm : Form
{
private string[] lotteryItems; // 用于存储抽奖项的数组
public MainForm()
{
InitializeComponent();
// 初始化下拉列表项
comboBox1.Items.AddRange(new string[] { "选项1", "选项2", "选项3", "选项4" });
lotteryItems = comboBox1.Items.Cast<string>().ToArray();
}
private void button1_Click(object sender, EventArgs e)
{
if (comboBox1.SelectedIndex != -1)
{
// 随机选择一个索引
int selectedIndex = new Random().Next(0, lotteryItems.Length);
// 显示在标签上
label1.Text = "中奖者:" + lotteryItems[selectedIndex];
}
else
{
label1.Text = "请选择一个抽奖选项";
}
}
}
}
```
在这段代码中,`comboBox1`是ComboBox控件的名称,`button1`是Button控件的名称,而`label1`是Label控件的名称。这段代码会根据ComboBox中选中的项,随机抽取一个结果并显示在Label上。
阅读全文
相关推荐
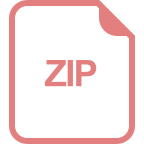
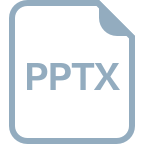
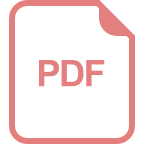















