当前工作目录下有一个文件名为class_score.txt的文本文件,存放着某班学生的姓名(第1列)、数学课成绩(第2列)和语文课成绩(第3列),每列数据用制表符(\t)进行分隔,文件内容如下所示: 请编程完成下列要求: (1)分别求这个班数学和语文的平均分(保留1位小数)并输出。 (2)找出两门课都不及格(<60)的学生,输出他们的学号和各科成绩。 (3)找出两门课的平均分在90分以上的学生,输出他们的学号和各科成绩。建议用三个函数分别实现以上要求。
时间: 2024-01-17 21:05:25 浏览: 82
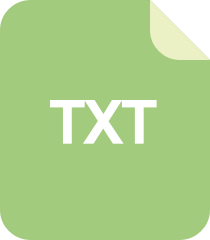
统计班级成绩.txt
以下是Python的代码实现:
```python
def read_file(file_name):
"""读取文件并返回学生信息列表"""
with open(file_name, 'r') as f:
lines = f.readlines()
students = []
for line in lines:
fields = line.strip().split('\t')
student = {'name': fields[0], 'math_score': int(fields[1]), 'chinese_score': int(fields[2])}
students.append(student)
return students
def calc_average_score(students, subject):
"""计算某个科目的平均分"""
scores = [s[subject+'_score'] for s in students]
return round(sum(scores) / len(students), 1)
def find_failed_students(students):
"""查找两门课都不及格的学生"""
failed_students = []
for student in students:
if student['math_score'] < 60 and student['chinese_score'] < 60:
failed_students.append(student)
return failed_students
def find_excellent_students(students):
"""查找两门课平均分在90分以上的学生"""
excellent_students = []
for student in students:
avg_score = (student['math_score'] + student['chinese_score']) / 2
if avg_score >= 90:
excellent_students.append(student)
return excellent_students
# 读取文件并获取学生信息列表
students = read_file('class_score.txt')
# 计算平均分并输出
math_avg_score = calc_average_score(students, 'math')
chinese_avg_score = calc_average_score(students, 'chinese')
print('数学平均分:', math_avg_score)
print('语文平均分:', chinese_avg_score)
# 查找两门课都不及格的学生并输出
failed_students = find_failed_students(students)
if failed_students:
print('两门课都不及格的学生:')
for student in failed_students:
print(student['name'], student['math_score'], student['chinese_score'])
else:
print('没有两门课都不及格的学生')
# 查找两门课平均分在90分以上的学生并输出
excellent_students = find_excellent_students(students)
if excellent_students:
print('两门课平均分在90分以上的学生:')
for student in excellent_students:
print(student['name'], student['math_score'], student['chinese_score'])
else:
print('没有两门课平均分在90分以上的学生')
```
其中,`read_file` 函数用于读取文件并返回学生信息列表;`calc_average_score` 函数用于计算某个科目的平均分;`find_failed_students` 函数用于查找两门课都不及格的学生;`find_excellent_students` 函数用于查找两门课平均分在90分以上的学生。最后,我们可以调用这些函数来完成题目要求。
阅读全文
相关推荐
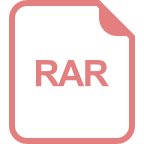
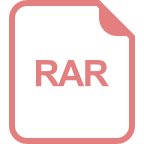






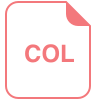
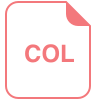
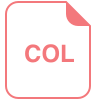
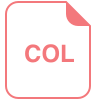

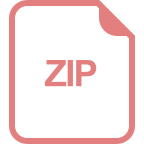
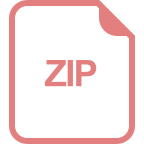