设计矩形Rect类,有两个float类属性成员长和宽,求面积和周长。 要求:设计两个以上的构造方法 (1)无参数,采用默认值0; (2)两个参数, 设计测试类,输入长与宽,利用这组教据定义对象1,输出对象1的面积和周长·利用无参构造方法定义对象2,将对象2的长与宽设置为15和6,输出对象2的面积和周长·结果不需要限定小数位数 【输入形式]对象1的长和宽 【输出形式】 对象1的面积和周长 对象2的面积和周长
时间: 2023-06-13 14:06:24 浏览: 62
下面是设计的矩形Rect类的代码实现:
```java
public class Rect {
private float length;
private float width;
public Rect() {
this.length = 0;
this.width = 0;
}
public Rect(float length, float width) {
this.length = length;
this.width = width;
}
public float getLength() {
return length;
}
public void setLength(float length) {
this.length = length;
}
public float getWidth() {
return width;
}
public void setWidth(float width) {
this.width = width;
}
public float getArea() {
return length * width;
}
public float getPerimeter() {
return 2 * (length + width);
}
}
```
下面是测试类的代码实现:
```java
public class RectTest {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
float length = sc.nextFloat();
float width = sc.nextFloat();
Rect rect1 = new Rect(length, width);
System.out.println("Rect1's area is " + rect1.getArea());
System.out.println("Rect1's perimeter is " + rect1.getPerimeter());
Rect rect2 = new Rect();
rect2.setLength(15);
rect2.setWidth(6);
System.out.println("Rect2's area is " + rect2.getArea());
System.out.println("Rect2's perimeter is " + rect2.getPerimeter());
}
}
```
在程序运行时,先输入对象1的长和宽,然后按照要求输出对象1和对象2的面积和周长。例如,输入长为10,宽为5,则输出如下:
```
10 5
Rect1's area is 50.0
Rect1's perimeter is 30.0
Rect2's area is 90.0
Rect2's perimeter is 42.0
```
注意,结果不需要限定小数位数。
相关推荐
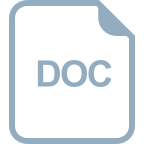
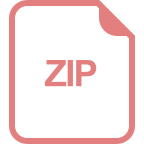
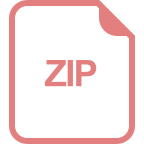















