python深度图转点云图并可视化示例代码
时间: 2023-09-06 09:05:23 浏览: 134
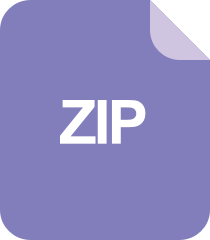
02 Halcon 深度图转点云图.zip

### 回答1:
很好,这是一个关于Python编程的问题,可以进行回答。以下是一个基于Open3D库的示例代码:
```python
import open3d as o3d
import numpy as np
# 读取深度图
depth_image = o3d.io.read_image('depth.png')
depth_array = np.asarray(depth_image)
# 内参矩阵
intrinsic = o3d.camera.PinholeCameraIntrinsic()
intrinsic.set_intrinsics(depth_array.shape[1], depth_array.shape[0], fx, fy, cx, cy)
# 将深度图转换为点云
point_cloud = o3d.geometry.PointCloud.create_from_depth_image(depth_image, intrinsic)
# 可视化
o3d.visualization.draw_geometries([point_cloud])
```
注意:要替换代码中的 fx, fy, cx, cy 值为你深度图对应的内参矩阵的值。
### 回答2:
Python深度图转点云图并可视化示例代码如下:
```python
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
def depth_to_pointcloud(depth_image, camera_intrinsics):
# 获取深度图的尺寸
height, width = depth_image.shape
# 获取相机参数,包括焦距、像素中心等
fx, fy = camera_intrinsics['fx'], camera_intrinsics['fy']
cx, cy = camera_intrinsics['cx'], camera_intrinsics['cy']
# 创建点云图
pointcloud = []
# 遍历深度图的每个像素
for v in range(height):
for u in range(width):
# 获取当前像素的深度值
depth = depth_image[v, u]
# 如果深度值有效,则计算对应的三维坐标
if depth > 0:
x = (u - cx) * depth / fx
y = (v - cy) * depth / fy
z = depth
# 添加三维坐标到点云图中
pointcloud.append([x, y, z])
# 将点云图转换为NumPy数组
pointcloud = np.array(pointcloud)
return pointcloud
# 深度图示例(假设为128x128的灰度图)
depth_image = np.random.rand(128, 128)
# 相机参数示例
camera_intrinsics = {'fx': 500, 'fy': 500, 'cx': 64, 'cy': 64}
# 转换深度图为点云图
pointcloud = depth_to_pointcloud(depth_image, camera_intrinsics)
# 可视化点云图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(pointcloud[:, 0], pointcloud[:, 1], pointcloud[:, 2], c='b', marker='.')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
```
以上代码实现了将一个假设为128x128像素的深度图转换为点云图,并使用Matplotlib绘制出点云图的三维可视化。先定义了一个函数`depth_to_pointcloud`,用于将深度图转换为点云图。然后通过随机生成的深度图和相机参数示例调用该函数,得到转换后的点云图。最后使用`matplotlib.pyplot`模块创建一个三维坐标系,并在坐标系中绘制点云图。
### 回答3:
下面是一个将深度图转换为点云图并可视化的示例代码:
```python
import numpy as np
import cv2
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
# 读取深度图
depth_image = cv2.imread('depth_image.png', cv2.IMREAD_GRAYSCALE)
# 将深度图转换为点云
fx = 525.0 # x方向的焦距
fy = 525.0 # y方向的焦距
cx = 319.5 # x方向的光心
cy = 239.5 # y方向的光心
rows, cols = depth_image.shape
points = []
for u in range(rows):
for v in range(cols):
depth = depth_image[u, v]
if depth > 0:
x = (v - cx) * depth / fx
y = (u - cy) * depth / fy
z = depth
points.append([x, y, z])
points = np.array(points)
# 可视化点云
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(points[:, 0], points[:, 1], points[:, 2], c='b', marker='o')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
```
以上代码假设你已经有名为 `depth_image.png` 的深度图,其中的像素值表示对应点的深度信息。代码会根据深度图的像素值计算出对应点的三维坐标,并用蓝色的小圆点表示在三维空间中的位置。你可以根据自己的实际深度图进行相应的修改,并运行代码来查看生成的点云图。
阅读全文
相关推荐
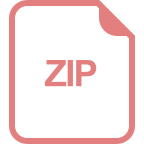




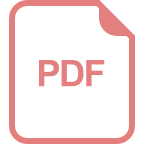
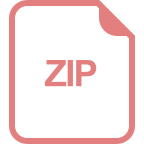
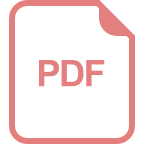
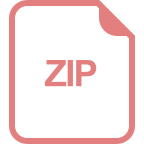
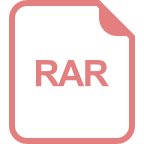
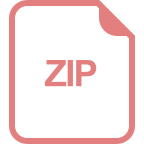
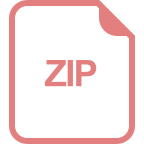
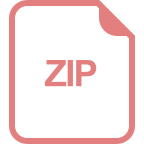
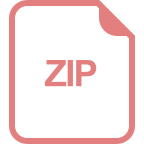