QFpt上传文件夹,并且上传此文件夹中还包含文件夹
时间: 2023-08-13 13:05:41 浏览: 163
QFtp类本身并不支持直接上传整个文件夹,但你可以通过递归遍历文件夹中的文件和子文件夹来实现上传整个文件夹的功能。以下是一个示例代码,演示如何使用QFtp上传文件夹及其子文件夹中的文件:
```cpp
#include <QtNetwork/QFtp>
#include <QtCore/QDir>
#include <QDebug>
void uploadFolder(QFtp &ftp, const QString &localFolderPath, const QString &remoteFolderPath)
{
QDir localDir(localFolderPath);
QStringList files = localDir.entryList(QDir::Files);
// 上传当前文件夹中的文件
for (const QString &fileName : files) {
QString localFilePath = localFolderPath + "/" + fileName;
QString remoteFilePath = remoteFolderPath + "/" + fileName;
ftp.put(localFilePath, remoteFilePath);
}
// 递归上传子文件夹
QStringList folders = localDir.entryList(QDir::Dirs | QDir::NoDotAndDotDot);
for (const QString &folderName : folders) {
QString localSubFolderPath = localFolderPath + "/" + folderName;
QString remoteSubFolderPath = remoteFolderPath + "/" + folderName;
// 在FTP服务器上创建对应的子文件夹
ftp.mkdir(remoteSubFolderPath);
ftp.cd(remoteSubFolderPath);
// 递归上传子文件夹中的文件
uploadFolder(ftp, localSubFolderPath, remoteSubFolderPath);
// 返回上一级目录
ftp.cd("..");
}
}
int main()
{
QFtp ftp;
// ... 连接FTP服务器等操作 ...
QString localFolder = "/path/to/local/folder";
QString remoteFolder = "/path/to/remote/folder";
// 递归上传文件夹
uploadFolder(ftp, localFolder, remoteFolder);
// 监听上传完成信号
QObject::connect(&ftp, &QFtp::done, [&](bool error) {
if (error) {
qDebug() << "上传文件夹失败:" << ftp.errorString();
} else {
qDebug() << "上传文件夹成功!";
}
});
// ... 其他操作 ...
return 0;
}
```
以上示例中的代码片段演示了如何使用递归函数`uploadFolder()`上传整个文件夹及其子文件夹中的文件。你需要通过修改`localFolder`和`remoteFolder`变量来指定本地文件夹路径和要上传到的FTP服务器上的目标文件夹路径。在递归函数中,我们首先获取当前文件夹中的文件列表,并分别上传这些文件。然后,获取当前文件夹中的子文件夹列表,并递归调用`uploadFolder()`函数来上传子文件夹中的文件。
需要注意的是,以上示例只是一个简单的演示,实际使用中可能需要处理更多的错误情况和异常情况,比如创建目录、切换目录等操作失败的情况。另外,还需要确保已经成功连接到FTP服务器并已经登录才能执行文件夹上传操作。
阅读全文
相关推荐
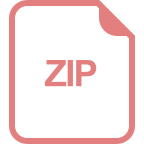
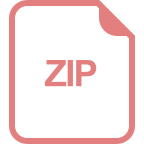
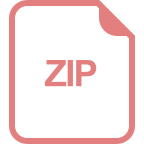
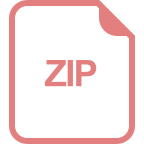
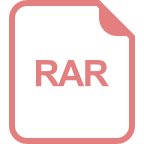
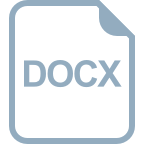
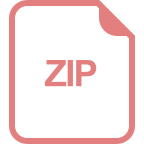
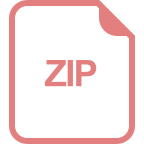
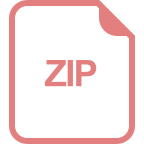
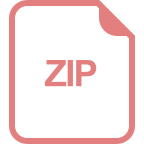
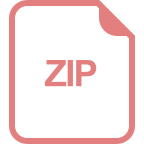
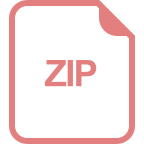
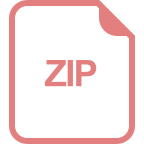
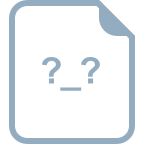
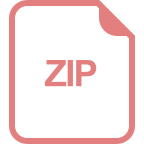
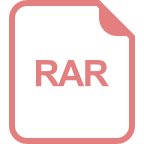