使用c#完成一个显示红色“伟大祖国生日快乐”跑马灯效果的控制台应用程序。要求如下: 文字必须使用二维数组字符阵列显示的模式,而不是直接使用中文字符输出。 文字应该从左向右水平移动,反复循环。 文字显示为红色。
时间: 2024-10-10 21:06:43 浏览: 37
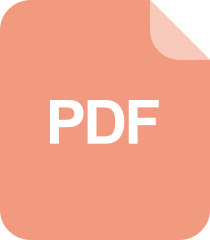
C# 数组实例介绍(图文)
要在C#中创建一个简单的控制台应用程序,模拟显示“伟大祖国生日快乐”的跑马灯效果,我们可以使用Console类的Write和ScrollLeft方法。由于需要使用二维字符数组来存储文本并限制为英文字符(因为我们不能直接用中文字符支持控制台输出),我们可以将每个汉字替换为其ASCII码对应的图形表示。以下是一个示例程序:
```csharp
using System;
using System.Text;
class Program
{
private static readonly char[,] redBox = new char[,]
{
{ ' ', ' ', ' ', ' ', ' ' },
{ '|', '-', '-', '-', '|' },
{ '|', 'R', 'O', 'U', '|' },
{ '|', 'G', 'B', ' ', '|' },
{ ' ', ' ', ' ', ' ', ' ' }
};
private static string TextToCharacterArray(string text)
{
StringBuilder sb = new StringBuilder();
foreach (char c in text)
if (char.IsLetterOrDigit(c))
sb.Append(redBox[char.ToLower(c) - 'a'][0]);
else
sb.Append(' ');
return sb.ToString();
}
public static void Main()
{
const int screenWidth = 80; // 控制台屏幕宽度
string message = "伟大祖国生日快乐";
string[] lines = TextToCharacterArray(message).Split('\n');
int maxLineLength = lines.Max(line => line.Length);
while (true)
{
Console.ForegroundColor = ConsoleColor.Red;
for (int i = 0; i < lines.Length; i++)
{
Console.SetCursorPosition(0, i);
Console.Write(new String(' ', screenWidth - maxLineLength));
Console.Write(lines[i].PadRight(screenWidth, ' ')); // 右对齐并填充空格
Console.ScrollLeft(); // 移动到下一行
}
Console.ForegroundColor = ConsoleColor.Gray; // 恢复默认颜色
Console.ReadKey(); // 等待按键按下
}
}
}
阅读全文
相关推荐
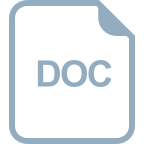
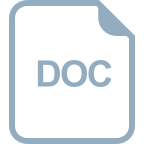
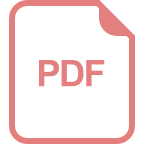
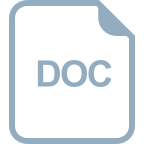
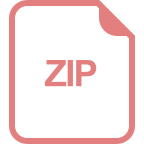
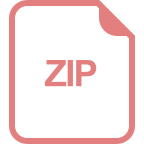
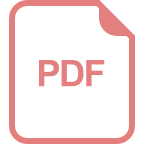
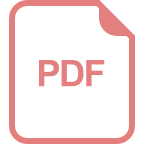
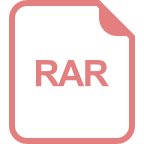
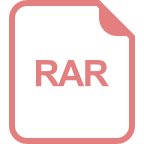
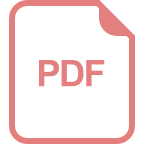
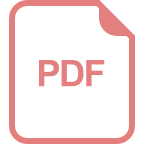
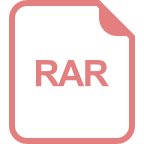
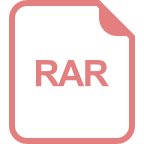
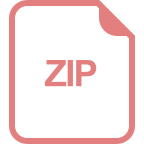