springboot配置线程池使用多线程插入数据
时间: 2023-07-23 10:27:57 浏览: 179
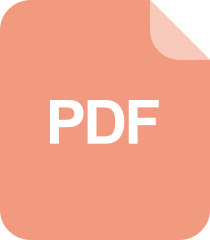
性能爆表:SpringBoot利用ThreadPoolTaskExecutor批量插入百万级数据实测
好的,针对您的问题,我可以给您提供以下的代码片段作为参考:
首先,您需要在您的 Spring Boot 项目中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
```
然后,在您的应用程序配置文件(例如 `application.properties` 或 `application.yml`)中添加以下配置:
```yaml
spring.datasource.url=jdbc:mysql://localhost:3306/testdb
spring.datasource.username=root
spring.datasource.password=123456
# 线程池配置
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.idle-timeout=60000
spring.datasource.hikari.pool-name=MyHikariCP
```
在您的代码中,您可以使用 `JdbcTemplate` 来执行数据库操作,并使用 `ThreadPoolTaskExecutor` 来并发地执行多个任务。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;
import javax.sql.DataSource;
@Configuration
public class AppConfig {
@Autowired
private DataSource dataSource;
@Bean
public JdbcTemplate jdbcTemplate() {
return new JdbcTemplate(dataSource);
}
@Bean
public ThreadPoolTaskExecutor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(25);
executor.setThreadNamePrefix("MyThread-");
executor.initialize();
return executor;
}
}
```
然后,您可以编写一个方法来并发地执行多个任务,例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Autowired
private JdbcTemplate jdbcTemplate;
@Autowired
private ThreadPoolTaskExecutor taskExecutor;
public void insertDataConcurrently() {
for (int i = 0; i < 10; i++) {
taskExecutor.execute(() -> {
jdbcTemplate.update("insert into my_table (column1, column2) values (?, ?)", "value1", "value2");
});
}
}
}
```
在上述代码中,我们使用 `taskExecutor.execute()` 方法来提交一个任务,这个任务会被分配到线程池中的一个线程来执行。每个任务都会执行一个插入操作,将一行数据插入到数据库表中。
希望这个代码片段能够对您有所帮助!
阅读全文
相关推荐
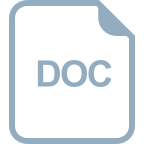
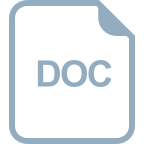
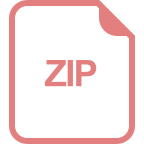
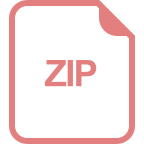
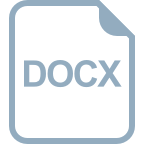
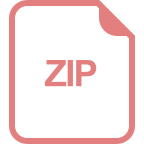
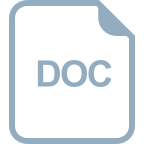
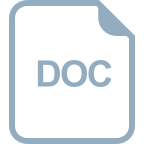
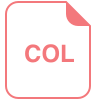
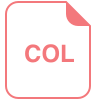
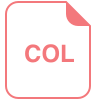
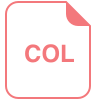





