stompClient.subscribe 参数使用说明
时间: 2023-11-18 13:04:56 浏览: 116
stompClient.subscribe方法用于订阅消息,接收特定目的地(队列或主题)上的消息。它可以通过以下参数进行配置:
1. destination:表示要订阅的目的地,可以是一个队列(queue)或者主题(topic)的名称。
2. headers:表示订阅消息时的头部信息,以键值对的形式提供。例如,可以指定订阅的持久性、过滤条件等。
3. callback:表示接收到消息时的回调函数。当有新消息到达时,会调用该回调函数来处理接收到的消息。
使用subscribe方法时,需要提供目的地和回调函数,以便监听并处理接收到的消息。可以通过headers参数来进一步设置订阅的属性和过滤条件。
例如,可以使用以下方式进行订阅:
```python
stompClient.subscribe(destination='/queue/my_queue', headers={'id': 'subscription-id'}, callback=handle_message)
```
其中,destination参数指定了要订阅的队列或主题名称,headers参数指定了订阅的属性,callback参数指定了处理接收到消息的回调函数handle_message。在回调函数中,你可以自定义处理接收到的消息的逻辑。
相关问题
代码详细说明stomp+websocket+springboot
好的,我可以为您详细说明一下stomp+websocket+springboot的实现方式。
首先,stomp是基于WebSocket协议的一种消息传输协议。它提供了一种简单的方式来实现消息传输,使得客户端和服务器之间可以通过WebSocket进行双向通信。而Spring Boot是一个快速开发Web应用程序的框架,它提供了对WebSocket和STOMP的内置支持,使得我们可以轻松地实现基于WebSocket和STOMP的应用程序。
下面是stomp+websocket+springboot的实现步骤:
1. 添加依赖
首先,在Spring Boot项目中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 配置WebSocket
在Spring Boot项目中,我们需要配置WebSocket。我们可以创建一个WebSocketConfig类,用来配置WebSocket相关的参数。在WebSocketConfig类中,我们需要覆盖registerStompEndpoints方法,该方法用于注册一个或多个STOMP端点,以便客户端可以连接到我们的WebSocket服务器。
```java
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/websocket").withSockJS();
}
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setApplicationDestinationPrefixes("/app");
}
}
```
在上面的代码中,我们注册了一个名为“/websocket”的STOMP端点,并启用了SockJS协议。这意味着我们的应用程序将支持基于WebSocket的连接,以及使用SockJS的WebSocket连接。
3. 消息处理
在WebSocketConfig类中,我们还需要配置消息处理。我们可以使用@MessageMapping注解来处理从客户端发送的消息,@SendTo注解将处理结果发送回客户端。例如:
```java
@Controller
public class WebSocketController {
@MessageMapping("/hello")
@SendTo("/topic/greetings")
public Greeting greeting(HelloMessage message) throws Exception {
Thread.sleep(1000);
return new Greeting("Hello, " + message.getName() + "!");
}
}
```
在上面的代码中,我们定义了一个名为“/hello”的消息映射,该映射将处理从客户端发送的消息。我们使用@SendTo注解将处理结果发送回客户端。
4. 客户端代码
最后,我们需要编写客户端代码来连接WebSocket服务器,并发送和接收消息。我们可以使用JavaScript或Java等语言编写客户端代码。以下是一个使用JavaScript编写的简单客户端代码示例:
```javascript
var stompClient = null;
function connect() {
var socket = new SockJS('/websocket');
stompClient = Stomp.over(socket);
stompClient.connect({}, function (frame) {
console.log('Connected: ' + frame);
stompClient.subscribe('/topic/greetings', function (greeting) {
showGreeting(JSON.parse(greeting.body).content);
});
});
}
function sendName() {
var name = document.getElementById('name').value;
stompClient.send("/app/hello", {}, JSON.stringify({ 'name': name }));
}
function showGreeting(message) {
var p = document.createElement('p');
p.appendChild(document.createTextNode(message));
document.getElementById('greetings').appendChild(p);
}
```
在上面的代码中,我们使用SockJS和Stomp.js库来连接WebSocket服务器,并发送和接收消息。我们使用subscribe方法来订阅指定的STOMP端点,并使用send方法来发送消息。
总结
以上就是stomp+websocket+springboot的实现方式。通过以上步骤,我们可以轻松地实现基于WebSocket和STOMP的应用程序,实现客户端和服务器之间的双向通信。
阅读全文
相关推荐
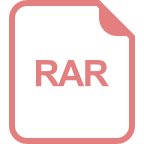
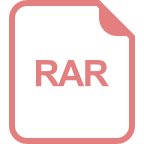
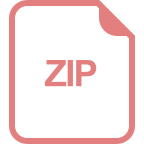
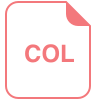
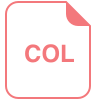
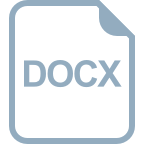
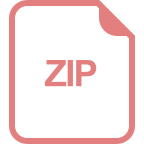
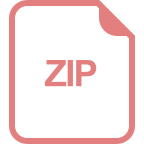