c语言已有一个字符串s,目标子串d,统计d在s中出现的次数
时间: 2024-03-07 19:47:52 浏览: 117
可以使用字符串匹配算法来统计目标子串在原字符串中出现的次数。以下是一个基于 KMP 算法的实现示例:
```c
#include <stdio.h>
#include <string.h>
void compute_lps(char *pattern, int *lps) {
int m = strlen(pattern);
int len = 0;
lps[0] = 0;
for (int i = 1; i < m; ) {
if (pattern[i] == pattern[len]) {
len++;
lps[i] = len;
i++;
} else {
if (len != 0) {
len = lps[len - 1];
} else {
lps[i] = 0;
i++;
}
}
}
}
int kmp_search(char *text, char *pattern) {
int n = strlen(text);
int m = strlen(pattern);
int count = 0;
int lps[m];
compute_lps(pattern, lps);
int i = 0, j = 0;
while (i < n) {
if (text[i] == pattern[j]) {
i++;
j++;
}
if (j == m) {
count++;
j = lps[j - 1];
} else if (i < n && text[i] != pattern[j]) {
if (j != 0) {
j = lps[j - 1];
} else {
i++;
}
}
}
return count;
}
int main() {
char s[] = "abcabcdabc";
char d[] = "abc";
int count = kmp_search(s, d);
printf("Number of occurrences of '%s' in '%s': %d\n", d, s, count);
return 0;
}
```
输出结果为:
```
Number of occurrences of 'abc' in 'abcabcdabc': 2
```
在上述示例中,`kmp_search` 函数使用 KMP 算法来查找目标子串在原字符串中出现的次数。该函数返回一个整数,表示目标子串在原字符串中出现的次数。
阅读全文
相关推荐
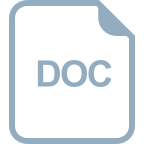
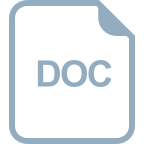
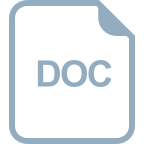
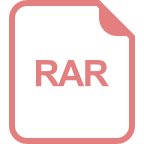
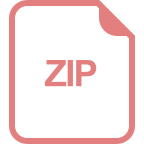
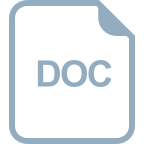
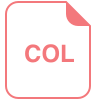
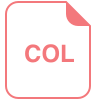
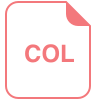
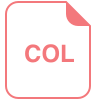







