map<string, uint> m_BoneMapping if (m_BoneMapping.find(NodeName) != m_BoneMapping.end()) { uint BoneIndex = m_BoneMapping[NodeName]; m_BoneInfo[BoneIndex].FinalTransformation = m_GlobalInverseTransform * GlobalTransformation * m_BoneInfo[BoneIndex].BoneOffset; }转换成Qt
时间: 2023-11-27 09:51:25 浏览: 39
这段代码是使用C++ STL中的map容器进行查找和映射,将一个字符串类型的骨骼名称(NodeName)对应到一个unsigned int类型的骨骼索引(BoneIndex)。然后根据骨骼索引,计算出这个骨骼的最终变换矩阵(FinalTransformation),并保存到m_BoneInfo数组中。
在Qt中,我们可以使用QMap类来代替STL中的map容器。QMap类提供了类似STL中map的键值对映射功能,但是其在插入和查找元素时具有更好的性能。
下面是将上述代码转换成Qt中使用QMap容器的代码示例:
```
QMap<QString, uint> m_BoneMapping;
if (m_BoneMapping.contains(NodeName)) {
uint BoneIndex = m_BoneMapping[NodeName];
m_BoneInfo[BoneIndex].FinalTransformation = m_GlobalInverseTransform * GlobalTransformation * m_BoneInfo[BoneIndex].BoneOffset;
}
```
注意,由于Qt中的QString类型与C++中的std::string类型不同,因此需要将map容器中的键类型从std::string改为QString,以便与QMap容器兼容。
相关问题
map<string,uint> m_BoneMapping ;m_BoneMapping.find(BoneName) == m_BoneMapping.end();转换成Qt
将map<string,uint> m_BoneMapping转换成QMap<QString, uint> m_BoneMapping,将std::string类型的BoneName替换为QString类型的BoneName,可以使用以下代码在Qt中实现查找:
```c++
#include <QMap>
#include <QString>
QMap<QString, uint> m_BoneMapping;
QString BoneName = "example";
if(m_BoneMapping.find(BoneName) == m_BoneMapping.end())
{
// BoneName 不存在于 m_BoneMapping 中
}
else
{
// BoneName 存在于 m_BoneMapping 中
}
```
使用QMap的find函数查找键值对时,需要将要查找的键值对的键(即BoneName)转换为QString类型。
map<string,uint> m_BoneMapping;转换成Qt
可以将map<string,uint>转换成QMap<QString, uint>,将std::string替换为QString,将std::map替换为QMap,如下所示:
```c++
#include <QMap>
#include <QString>
QMap<QString, uint> m_BoneMapping;
```
使用QMap与使用std::map的方式类似,可以使用insert、find等函数对键值对进行存储和查找。需要注意的是,QString与std::string在一些细节上有所不同,需要注意转换。
相关推荐
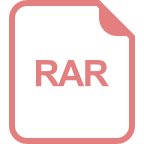













